设置一个父类phone,座机类,移动电话类,测试类为继承关系,,座机类和移动手机类都继承自一个通信设备类,通信设备类包含通信设备的一些基本属性和方法,比如设备编号、设备状态、拨打电话、接收电话等。座机类和移动手机类分别添加一些特定的属性和方法。 座机类的特定属性包括:座机号码、座机类型(家用、办公室等)、座机费用等。座机类的特定方法包括:计算座机电话费用、查询余额和充话费等。 移动手机类的特定属性包括:手机号码、所属运营商、通话时长、移动手机费用等。移动手机类的特定方法包括:计算移动电话费用、查询余额和充话费等。 测试类主要用来测试座机类和移动手机类的功能,包括拨打电话、接收电话、查询余额、充话费等操作。 java实现,输出设备编号、设备状态、拨打电话、接收电话,座机号码、座机类型(家用、办公室等)、座机费用,拨打电话、接收电话、查询余额、充话费
时间: 2023-12-11 12:03:55 浏览: 26
好的,以下是Java代码实现:
```java
// 通信设备类
class CommunicationDevice {
String deviceId; // 设备编号
boolean deviceStatus; // 设备状态
// 拨打电话方法
void makeCall(String phoneNumber) {
// 实现拨打电话的逻辑
}
// 接收电话方法
void receiveCall(String phoneNumber) {
// 实现接收电话的逻辑
}
}
// 座机类
class LandlinePhone extends CommunicationDevice {
String phoneNumber; // 座机号码
String phoneType; // 座机类型(家用、办公室等)
double phoneCost; // 座机费用
// 计算座机电话费用方法
double calculatePhoneCost() {
// 实现计算座机电话费用的逻辑
return phoneCost;
}
// 查询余额方法
double checkBalance() {
// 实现查询余额的逻辑
return 0.0;
}
// 充话费方法
void recharge(double amount) {
// 实现充话费的逻辑
}
}
// 移动电话类
class MobilePhone extends CommunicationDevice {
String phoneNumber; // 手机号码
String carrier; // 所属运营商
double callDuration; // 通话时长
double phoneCost; // 移动电话费用
// 计算移动电话费用方法
double calculatePhoneCost() {
// 实现计算移动电话费用的逻辑
return phoneCost;
}
// 查询余额方法
double checkBalance() {
// 实现查询余额的逻辑
return 0.0;
}
// 充话费方法
void recharge(double amount) {
// 实现充话费的逻辑
}
}
// 测试类
public class Test {
public static void main(String[] args) {
// 创建座机对象并测试
LandlinePhone landlinePhone = new LandlinePhone();
landlinePhone.deviceId = "001";
landlinePhone.deviceStatus = true;
landlinePhone.phoneNumber = "12345678";
landlinePhone.phoneType = "家用";
landlinePhone.phoneCost = 0.2;
System.out.println("设备编号:" + landlinePhone.deviceId);
System.out.println("设备状态:" + landlinePhone.deviceStatus);
landlinePhone.makeCall("87654321");
landlinePhone.receiveCall("87654321");
System.out.println("座机号码:" + landlinePhone.phoneNumber);
System.out.println("座机类型:" + landlinePhone.phoneType);
System.out.println("座机费用:" + landlinePhone.calculatePhoneCost());
System.out.println("余额:" + landlinePhone.checkBalance());
landlinePhone.recharge(100.0);
// 创建移动电话对象并测试
MobilePhone mobilePhone = new MobilePhone();
mobilePhone.deviceId = "002";
mobilePhone.deviceStatus = true;
mobilePhone.phoneNumber = "13888888888";
mobilePhone.carrier = "中国移动";
mobilePhone.callDuration = 30.0;
mobilePhone.phoneCost = 0.5;
System.out.println("设备编号:" + mobilePhone.deviceId);
System.out.println("设备状态:" + mobilePhone.deviceStatus);
mobilePhone.makeCall("13999999999");
mobilePhone.receiveCall("13999999999");
System.out.println("手机号码:" + mobilePhone.phoneNumber);
System.out.println("所属运营商:" + mobilePhone.carrier);
System.out.println("通话时长:" + mobilePhone.callDuration);
System.out.println("移动电话费用:" + mobilePhone.calculatePhoneCost());
System.out.println("余额:" + mobilePhone.checkBalance());
mobilePhone.recharge(50.0);
}
}
```
相关推荐
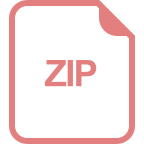
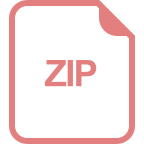














