android+应用程序+cpu使用率,计算Android中进程的CPU使用率
时间: 2023-12-19 14:04:01 浏览: 63
要计算Android中进程的CPU使用率,可以使用以下步骤:
1. 获取进程的CPU时间信息。可以使用`android.os.Process`类中的`getElapsedCpuTime()`方法获取进程的CPU时间信息。
2. 计算CPU时间差。在不同时间点获取进程的CPU时间信息,然后计算CPU时间差。
3. 计算CPU使用率。CPU使用率可以通过以下公式计算:
CPU使用率 = (CPU时间差 / 系统运行时间) * CPU核心数
其中,系统运行时间可以使用`android.os.SystemClock`类中的`uptimeMillis()`方法获取,CPU核心数可以使用`Runtime.getRuntime().availableProcessors()`方法获取。
以下是一个示例代码,可以计算指定进程的CPU使用率:
```java
import android.os.Process;
import android.os.SystemClock;
public class CpuUsageCalculator {
private static final int SAMPLE_TIME_MS = 1000; // 采样时间间隔,单位:毫秒
private static final int PROCESS_STATE_RUNNING = Process.PROCESS_STATE_RUNNING;
private static final int PROCESS_STATE_TOP = Process.PROCESS_STATE_TOP;
private int mPid;
private long mLastCpuTime;
private long mLastSampleTime;
public CpuUsageCalculator(int pid) {
mPid = pid;
mLastCpuTime = Process.getElapsedCpuTime();
mLastSampleTime = SystemClock.uptimeMillis();
}
public float getCpuUsage() {
long cpuTime = Process.getElapsedCpuTime();
long sampleTime = SystemClock.uptimeMillis();
long cpuTimeDiff = cpuTime - mLastCpuTime;
long sampleTimeDiff = sampleTime - mLastSampleTime;
// 计算CPU使用率
float cpuUsage = 0;
if (sampleTimeDiff > 0) {
int cpuCoreCount = Runtime.getRuntime().availableProcessors();
long systemTime = SystemClock.elapsedRealtime();
long systemTimeDiff = systemTime - mLastSampleTime;
float cpuUsagePercent = (float) cpuTimeDiff / (float) systemTimeDiff;
cpuUsage = cpuUsagePercent * cpuCoreCount;
}
// 更新状态
mLastCpuTime = cpuTime;
mLastSampleTime = sampleTime;
return cpuUsage;
}
public boolean isProcessRunning() {
int processState = Process.getProcessState(mPid);
return processState == PROCESS_STATE_RUNNING || processState == PROCESS_STATE_TOP;
}
}
```
使用示例:
```java
CpuUsageCalculator calculator = new CpuUsageCalculator(pid);
while (calculator.isProcessRunning()) {
float cpuUsage = calculator.getCpuUsage();
Log.d(TAG, "CPU usage: " + cpuUsage);
SystemClock.sleep(SAMPLE_TIME_MS);
}
```
相关推荐
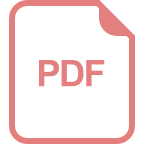
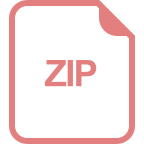














