在 Flask 中使用 SQLite 数据库,并且想要实现多个下拉式查询
时间: 2023-05-26 22:01:50 浏览: 63
可以使用 Flask-SQLAlchemy 和 WTForms 来实现多个下拉式查询。
首先,定义一个模型类来表示你的数据表,这里我们以一个简单的例子为例:
```python
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
class Fruit(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50))
color = db.Column(db.String(50))
```
然后,定义一个表单类(使用 WTForms),并且添加多个查询下拉列表:
```python
from wtforms import Form, SelectField
from .models import Fruit
class SearchForm(Form):
fruits = SelectField('Fruits', choices=[(str(fruit.id), fruit.name) for fruit in Fruit.query.all()])
colors = SelectField('Colors', choices=[('red', 'Red'), ('green', 'Green'), ('yellow', 'Yellow')])
```
最后,在你的视图函数中,处理表单提交数据并根据数据查询数据库:
```python
from flask import render_template, request
from .models import Fruit
from .forms import SearchForm
@app.route('/', methods=['GET', 'POST'])
def index():
form = SearchForm()
if form.validate_on_submit():
fruit_id = form.fruits.data
color = form.colors.data
fruits = Fruit.query.filter_by(id=fruit_id, color=color).all()
else:
fruits = Fruit.query.all()
return render_template('index.html', form=form, fruits=fruits)
```
在 HTML 模板文件中,你可以使用表单类中定义的下拉列表并且渲染查询结果:
```html
<form method="POST">
{{ form.fruits }}
{{ form.colors }}
<button type="submit">Search</button>
</form>
<ul>
{% for fruit in fruits %}
<li>{{ fruit.name }} - {{ fruit.color }}</li>
{% endfor %}
</ul>
```
相关推荐
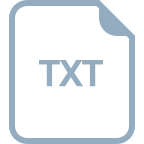
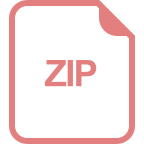
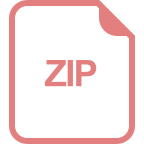
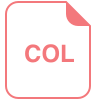
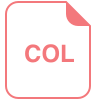
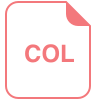
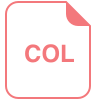










