定义一个Dog,包含name、age、sex和weight等属性以及对这些属性操作的方法。 要求用字符指针描述name,并且用对象指针来测试这个类。
时间: 2024-10-25 21:14:15 浏览: 36
好的,我可以帮你创建一个简单的C++类`Dog`来满足你的需求。首先,我们需要声明一个结构体来存储狗的基本信息:
```cpp
// 定义Dog类
struct Dog {
char* name; // 使用字符指针存储名字
int age;
char sex[2]; // 为了表示性别,这里假设最多只有'M'(雄性)和'F'(雌性)
float weight;
// 构造函数设置默认值
Dog(const char* _name = "", int _age = 0, const char _sex = 'M', float _weight = 0.0f) :
name(_name), age(_age), sex(_sex == 'M' ? "M" : "F"), weight(_weight) {}
// 拷贝构造函数和析构函数
Dog(const Dog& other) : Dog(other.name, other.age, other.sex, other.weight) {}
~Dog() { delete[] name; } // 释放name所占内存
// 方法来操作属性
void setName(const char* newName) { if (newName != nullptr) { delete[] name; name = new char[strlen(newName) + 1]; strcpy(name, newName); } }
int getAge() const { return age; }
char getSex() const { return sex[0]; }
float getWeight() const { return weight; }
// 朋友函数用于演示如何通过对象指针调用类方法
friend void displayInfo(Dog& dog);
};
// 显示狗的信息
void displayInfo(Dog& dog) {
std::cout << "Name: " << dog.name << ", Age: " << dog.getAge() << ", Sex: " << dog.getSex() << ", Weight: " << dog.getWeight() << std::endl;
}
```
在这个例子中,我们定义了一个`Dog`类,它有四个成员变量:`name`(字符指针)、`age`(整数)、`sex`(字符数组代表性别)和`weight`(浮点数)。还提供了相应的构造函数、拷贝构造函数、析构函数以及操作属性的方法。
然后,你可以通过一个`Dog`类型的对象指针来调用`displayInfo()`方法来显示狗狗的信息:
```cpp
int main() {
Dog myDog("Max", 3, 'M', 15.0f);
displayInfo(myDog);
// 创建另一个狗的对象并指向它
Dog anotherDog("Bella", 4, 'F', 20.0f);
displayInfo(anotherDog);
return 0;
}
```
阅读全文
相关推荐
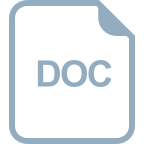
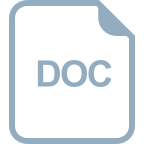
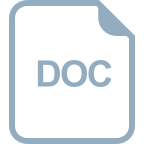















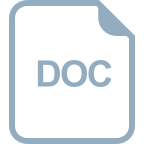