Java做出简单的计算器带有自定义布局,swing按钮
时间: 2023-12-08 17:05:22 浏览: 31
好的,下面是一个简单的Java计算器的代码,使用了Swing布局和按钮:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Calculator extends JFrame implements ActionListener {
private JTextField textField;
private JPanel buttonPanel;
public Calculator() {
super("Calculator");
// 创建文本框和按钮面板
textField = new JTextField();
buttonPanel = new JPanel(new GridLayout(4, 4));
// 添加按钮
addButton("7");
addButton("8");
addButton("9");
addButton("/");
addButton("4");
addButton("5");
addButton("6");
addButton("*");
addButton("1");
addButton("2");
addButton("3");
addButton("-");
addButton("0");
addButton(".");
addButton("C");
addButton("+");
// 设置布局
setLayout(new BorderLayout());
add(textField, BorderLayout.NORTH);
add(buttonPanel, BorderLayout.CENTER);
// 设置窗口属性
setSize(300, 300);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
// 添加按钮的方法
private void addButton(String label) {
JButton button = new JButton(label);
button.addActionListener(this);
buttonPanel.add(button);
}
// 处理按钮点击事件的方法
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
if (command.equals("C")) {
textField.setText("");
} else if (command.equals("=")) {
String expression = textField.getText();
try {
double result = evaluate(expression);
textField.setText(Double.toString(result));
} catch (Exception ex) {
textField.setText("Error");
}
} else {
textField.setText(textField.getText() + command);
}
}
// 计算表达式的方法
private double evaluate(String expression) throws Exception {
return new Object() {
int pos = -1, ch;
void nextChar() {
ch = (++pos < expression.length()) ? expression.charAt(pos) : -1;
}
boolean skip(char c) {
while (ch == ' ') nextChar();
if (ch == c) {
nextChar();
return true;
}
return false;
}
double parse() throws Exception {
nextChar();
double x = parseExpression();
if (pos < expression.length()) throw new Exception("Unexpected: " + (char)ch);
return x;
}
double parseExpression() throws Exception {
double x = parseTerm();
for (;;) {
if (skip('+')) x += parseTerm(); // addition
else if (skip('-')) x -= parseTerm(); // subtraction
else return x;
}
}
double parseTerm() throws Exception {
double x = parseFactor();
for (;;) {
if (skip('*')) x *= parseFactor(); // multiplication
else if (skip('/')) x /= parseFactor(); // division
else return x;
}
}
double parseFactor() throws Exception {
if (skip('+')) return parseFactor(); // unary plus
if (skip('-')) return -parseFactor(); // unary minus
double x;
int startPos = this.pos;
if (skip('(')) { // parentheses
x = parseExpression();
skip(')');
} else if ((ch >= '0' && ch <= '9') || ch == '.') { // numbers
while ((ch >= '0' && ch <= '9') || ch == '.') nextChar();
x = Double.parseDouble(expression.substring(startPos, this.pos));
} else if (ch >= 'a' && ch <= 'z') { // functions
while (ch >= 'a' && ch <= 'z') nextChar();
String func = expression.substring(startPos, this.pos);
x = parseFactor();
if (func.equals("sqrt")) x = Math.sqrt(x);
else if (func.equals("sin")) x = Math.sin(Math.toRadians(x));
else if (func.equals("cos")) x = Math.cos(Math.toRadians(x));
else if (func.equals("tan")) x = Math.tan(Math.toRadians(x));
else throw new Exception("Unknown function: " + func);
} else {
throw new Exception("Unexpected: " + (char)ch);
}
if (skip('^')) x = Math.pow(x, parseFactor()); // exponentiation
return x;
}
}.parse();
}
public static void main(String[] args) {
new Calculator();
}
}
```
这个计算器使用了一个中缀表达式求值算法来计算表达式的值。你可以根据自己的需要修改它的布局和按钮。
相关推荐
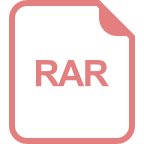
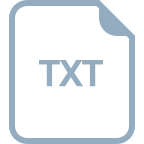
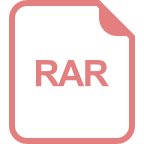














