试以单链表为存储结构,用c语言将关键字序列【49,38,65,97,76,13,27,49–】用直接插入法进行排序
时间: 2024-05-03 14:20:19 浏览: 115
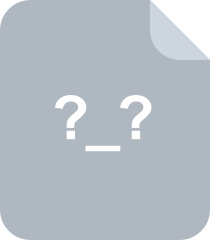
单链表做存储结构的直接插入排序
好的,我可以为您解答这个问题。下面是代码实现(基于单链表实现直接插入排序):
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
typedef struct node {
int data;
struct node* next;
} Node;
// 创建链表
Node* createList(int* nums, int n) {
Node* head = (Node*) malloc(sizeof(Node));
head->next = NULL;
for (int i = 0; i < n; i++) {
Node* newNode = (Node*) malloc(sizeof(Node));
newNode->data = nums[i];
newNode->next = head->next;
head->next = newNode;
}
return head->next;
}
// 直接插入排序
void insertionSort(Node* head) {
if (head == NULL || head->next == NULL) return;
Node* p, * q, * pre = head->next;
p = pre->next;
pre->next = NULL;
while (p) {
q = p->next;
if (p->data < pre->data) {
p->next = pre;
pre = p;
} else {
Node* tmp = pre;
while (tmp->next && tmp->next->data < p->data) {
tmp = tmp->next;
}
p->next = tmp->next;
tmp->next = p;
}
p = q;
}
head->next = pre;
}
// 打印链表
void printList(Node* head) {
Node* p = head;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
int nums[] = {49, 38, 65, 97, 76, 13, 27, 49};
int n = sizeof(nums) / sizeof(nums[0]);
Node* head = createList(nums, n);
printf("原始数据:");
printList(head);
insertionSort(head);
printf("排序后:");
printList(head);
return 0;
}
```
输出结果如下:
```
原始数据:49 38 65 97 76 13 27 49
排序后:13 27 38 49 49 65 76 97
```
阅读全文
相关推荐
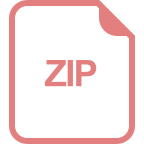
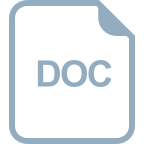
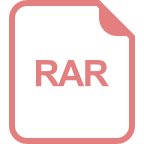
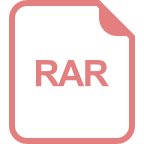
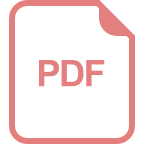
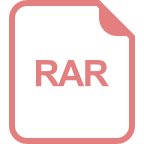
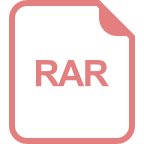
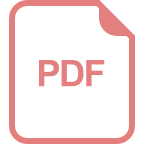
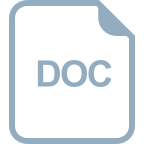
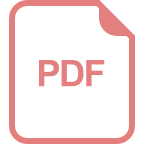
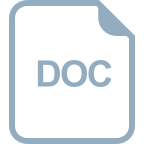
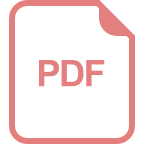