c语言迷宫游戏代码
时间: 2023-08-12 18:18:32 浏览: 80
好的,下面是一个简单的C语言迷宫游戏的代码示例,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_ROWS 10
#define MAX_COLS 10
char maze[MAX_ROWS][MAX_COLS] = {
{'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'},
{'#', '.', '.', '.', '#', '.', '.', '.', '.', '#'},
{'#', '.', '#', '.', '#', '.', '#', '#', '.', '#'},
{'#', '.', '#', '.', '.', '.', '.', '.', '.', '#'},
{'#', '.', '.', '#', '#', '.', '#', '#', '.', '#'},
{'#', '#', '.', '.', '#', '.', '.', '.', '.', '#'},
{'#', '.', '#', '.', '#', '#', '#', '.', '#', '#'},
{'#', '.', '.', '.', '.', '.', '.', '.', '.', '#'},
{'#', '#', '#', '#', '#', '#', '#', '#', '.', '#'},
{'#', '.', '.', '.', '.', '.', '.', '#', '#', '#'}
};
int start_row = 1;
int start_col = 1;
int end_row = 8;
int end_col = 8;
void print_maze() {
int row, col;
for (row = 0; row < MAX_ROWS; row++) {
for (col = 0; col < MAX_COLS; col++) {
printf("%c", maze[row][col]);
}
printf("\n");
}
}
int solve_maze(int row, int col) {
if (row == end_row && col == end_col) {
return 1;
}
if (maze[row][col] == '#' || maze[row][col] == '+') {
return 0;
}
maze[row][col] = '+';
if (solve_maze(row - 1, col) || solve_maze(row + 1, col) || solve_maze(row, col - 1) || solve_maze(row, col + 1)) {
return 1;
}
maze[row][col] = '.';
return 0;
}
int main() {
printf("Welcome to the maze game!\n");
printf("Press enter to start...");
getchar();
system("clear");
print_maze();
printf("Press enter to start solving the maze...");
getchar();
system("clear");
if (solve_maze(start_row, start_col)) {
printf("Congratulations! You have solved the maze!\n");
print_maze();
} else {
printf("Sorry, you have failed to solve the maze.\n");
}
return 0;
}
```
这个迷宫游戏使用二维数组来存储迷宫的地图,'#'表示墙壁,'.'表示通路,'+'表示已经探索过的位置。使用递归算法来搜索迷宫解决方案。在开始游戏前,需要指定起点和终点的位置。
相关推荐
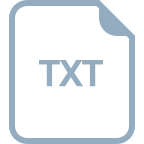
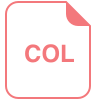
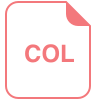












