vue3+ts实现小球抛物线
时间: 2023-08-29 19:06:58 浏览: 245
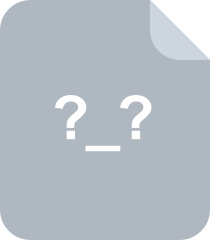
小球抛物线
首先,我们需要安装Vue 3和TypeScript的相关依赖。可以通过以下命令进行安装:
```
npm install vue@next vue-router@next @vue/compiler-sfc@next typescript
```
然后,在Vue 3项目中创建一个小球组件,该组件将接收起始坐标、目标坐标和抛物线高度作为属性。代码如下:
```html
<template>
<div class="ball" :style="{ top: y + 'px', left: x + 'px' }"></div>
</template>
<script lang="ts">
import { defineComponent, ref, watchEffect } from 'vue';
export default defineComponent({
props: {
startX: {
type: Number,
required: true,
},
startY: {
type: Number,
required: true,
},
endX: {
type: Number,
required: true,
},
endY: {
type: Number,
required: true,
},
height: {
type: Number,
required: true,
},
},
setup(props) {
const x = ref(props.startX);
const y = ref(props.startY);
const deltaX = props.endX - props.startX;
const deltaY = props.endY - props.startY;
const duration = Math.sqrt(deltaX ** 2 + deltaY ** 2) * 10;
const startTime = Date.now();
watchEffect(() => {
const currentTime = Date.now() - startTime;
const t = Math.min(1, currentTime / duration);
x.value = props.startX + deltaX * t;
y.value = props.startY + deltaY * t - ((4 * props.height * t * (1 - t)) / 1);
});
return {
x,
y,
};
},
});
</script>
<style>
.ball {
position: absolute;
width: 20px;
height: 20px;
border-radius: 50%;
background-color: red;
}
</style>
```
在该组件中,我们使用了Vue 3的Composition API来管理状态。我们定义了起始坐标和目标坐标作为必需的属性,并且使用`watchEffect`函数来监听这些属性的变化。在`watchEffect`回调函数中,我们计算小球的当前坐标,并将其设置为`x`和`y`变量的值。
我们使用以下公式计算小球的抛物线运动:
```
x = startX + deltaX * t
y = startY + deltaY * t - ((4 * height * t * (1 - t)) / 1)
```
其中,`startX`和`startY`是小球的起始坐标,`deltaX`和`deltaY`是小球的运动距离,`height`是小球的抛物线高度,`t`是小球的运动时间占总运动时间的比例。
最后,我们将小球的位置设置为`x`和`y`的值,并在样式中使用绝对定位来使其在页面上正确显示。
在父组件中,我们可以使用该组件并传递起始坐标、目标坐标和抛物线高度属性。例如:
```html
<template>
<div class="container">
<ball :startX="100" :startY="100" :endX="500" :endY="500" :height="100" />
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
import Ball from './Ball.vue';
export default defineComponent({
components: {
Ball,
},
});
</script>
<style>
.container {
position: relative;
width: 600px;
height: 600px;
border: 1px solid black;
}
</style>
```
这将在容器中创建一个小球,小球将从`(100, 100)`的位置开始,向`(500, 500)`的位置移动,并在高度为100的抛物线上运动。
阅读全文
相关推荐
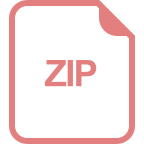
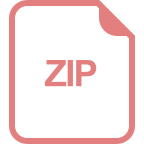
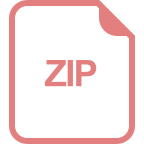
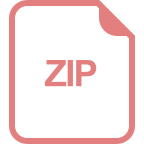
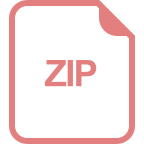
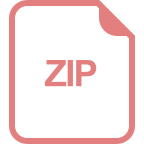
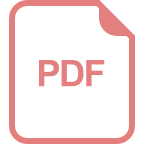
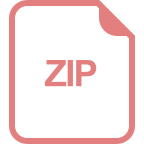
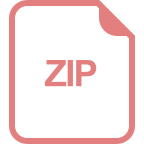
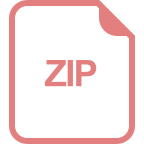
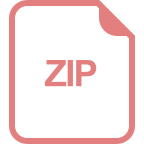
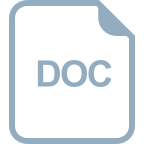
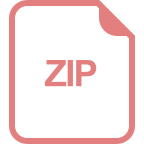
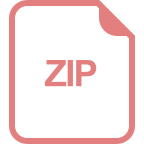
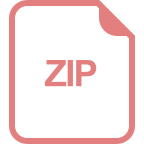