用c++写一个通讯录管理软件
时间: 2023-03-09 16:45:25 浏览: 166
好的,我可以用C语言来写一个通讯录管理软件。首先,我需要定义一个结构体来存储通讯录信息,例如联系人姓名、电话号码、地址等。然后,我需要实现功能,如添加、查找、删除等操作,使用者可以自由地管理通讯录条目。最后,我还可以实现一些附加功能,如导出通讯录到文本文件、从文本文件导入通讯录等。
相关问题
c++通讯录管理软件
以下是一个简单的C++通讯录管理软件的示例,它使用文件存储数据和链表操作数据。该程序可以添加、删除、查找和显示联系人信息。请注意,该程序仅供学习和参考,不得用于商业用途。
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 联系人结构体
struct Contact {
string name;
string phone;
string email;
};
// 联系人节点结构体
struct Node {
Contact data;
Node* next;
};
// 全局变量
Node* head = NULL; // 链表头指针
// 函数声明
void loadContacts(); // 从文件中加载联系人信息
void saveContacts(); // 将联系人信息保存到文件中
void addContact(); // 添加联系人
void deleteContact(); // 删除联系人
void searchContact(); // 查找联系人
void displayContacts(); // 显示所有联系人
int main() {
loadContacts(); // 加载联系人信息
int choice;
do {
// 显示菜单
cout << "请选择操作:" << endl;
cout << "1. 添加联系人" << endl;
cout << "2. 删除联系人" << endl;
cout << "3. 查找联系人" << endl;
cout << "4. 显示所有联系人" << endl;
cout << "5. 退出" << endl;
cin >> choice;
switch (choice) {
case 1:
addContact();
break;
case 2:
deleteContact();
break;
case 3:
searchContact();
break;
case 4:
displayContacts();
break;
case 5:
saveContacts(); // 保存联系人信息到文件
cout << "感谢使用!" << endl;
break;
default:
cout << "无效的选择,请重新输入。" << endl;
break;
}
} while (choice != 5);
return 0;
}
// 从文件中加载联系人信息
void loadContacts() {
ifstream infile("contacts.txt");
if (infile) {
while (!infile.eof()) {
Contact contact;
getline(infile, contact.name);
getline(infile, contact.phone);
getline(infile, contact.email);
Node* node = new Node;
node->data = contact;
node->next = head;
head = node;
}
infile.close();
}
}
// 将联系人信息保存到文件中
void saveContacts() {
ofstream outfile("contacts.txt");
if (outfile) {
Node* node = head;
while (node != NULL) {
outfile << node->data.name << endl;
outfile << node->data.phone << endl;
outfile << node->data.email << endl;
node = node->next;
}
outfile.close();
}
}
// 添加联系人
void addContact() {
Contact contact;
cout << "请输入联系人姓名:";
cin >> contact.name;
cout << "请输入联系人电话:";
cin >> contact.phone;
cout << "请输入联系人邮箱:";
cin >> contact.email;
Node* node = new Node;
node->data = contact;
node->next = head;
head = node;
cout << "联系人添加成功!" << endl;
}
// 删除联系人
void deleteContact() {
string name;
cout << "请输入要删除的联系人姓名:";
cin >> name;
Node* prev = NULL;
Node* curr = head;
while (curr != NULL) {
if (curr->data.name == name) {
if (prev == NULL) {
head = curr->next;
} else {
prev->next = curr->next;
}
delete curr;
cout << "联系人删除成功!" << endl;
return;
}
prev = curr;
curr = curr->next;
}
cout << "未找到该联系人!" << endl;
}
// 查找联系人
void searchContact() {
string name;
cout << "请输入要查找的联系人姓名:";
cin >> name;
Node* node = head;
while (node != NULL) {
if (node->data.name == name) {
cout << "联系人信息如下:" << endl;
cout << "姓名:" << node->data.name << endl;
cout << "电话:" << node->data.phone << endl;
cout << "邮箱:" << node->data.email << endl;
return;
}
node = node->next;
}
cout << "未找到该联系人!" << endl;
}
// 显示所有联系人
void displayContacts() {
Node* node = head;
while (node != NULL) {
cout << "姓名:" << node->data.name << endl;
cout << "电话:" << node->data.phone << endl;
cout << "邮箱:" << node->data.email << endl;
node = node->next;
}
}
--相关问题--:
阅读全文
相关推荐
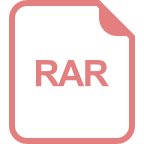


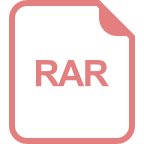

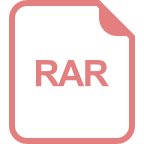








