给我一个 C# Npoi导出word excel 的helper
时间: 2024-02-13 18:00:31 浏览: 29
以下是一个示例 C# Npoi 导出 Word 和 Excel 的 Helper 类:
```csharp
using NPOI.HSSF.UserModel;
using NPOI.SS.UserModel;
using NPOI.XSSF.UserModel;
using NPOI.XWPF.UserModel;
using System.Collections.Generic;
using System.IO;
namespace MyProject.Helpers
{
public class NpoiHelper
{
/// <summary>
/// 导出 Excel
/// </summary>
/// <param name="data">数据</param>
/// <param name="filePath">文件路径</param>
public static bool ExportExcel(List<List<string>> data, string filePath)
{
IWorkbook workbook;
if (Path.GetExtension(filePath) == ".xls")
{
workbook = new HSSFWorkbook();
}
else
{
workbook = new XSSFWorkbook();
}
ISheet sheet = workbook.CreateSheet("Sheet1");
for (int i = 0; i < data.Count; i++)
{
IRow row = sheet.CreateRow(i);
for (int j = 0; j < data[i].Count; j++)
{
ICell cell = row.CreateCell(j);
cell.SetCellValue(data[i][j]);
}
}
using (FileStream stream = new FileStream(filePath, FileMode.Create, FileAccess.Write))
{
workbook.Write(stream);
}
return true;
}
/// <summary>
/// 导出 Word
/// </summary>
/// <param name="data">数据</param>
/// <param name="filePath">文件路径</param>
public static bool ExportWord(List<string> data, string filePath)
{
XWPFDocument doc = new XWPFDocument();
XWPFParagraph p = doc.CreateParagraph();
foreach (string s in data)
{
XWPFRun r = p.CreateRun();
r.SetText(s);
r.AddCarriageReturn();
}
using (FileStream stream = new FileStream(filePath, FileMode.Create, FileAccess.Write))
{
doc.Write(stream);
}
return true;
}
}
}
```
使用示例:
```csharp
// 导出 Excel
List<List<string>> data = new List<List<string>>()
{
new List<string> { "姓名", "年龄", "性别" },
new List<string> { "张三", "18", "男" },
new List<string> { "李四", "20", "女" }
};
NpoiHelper.ExportExcel(data, "D:\\test.xlsx");
// 导出 Word
List<string> data = new List<string>()
{
"这是一段文本。",
"这是另一段文本。"
};
NpoiHelper.ExportWord(data, "D:\\test.docx");
```
注意,使用该 Helper 类需要安装 NPOI NuGet 包。
相关推荐
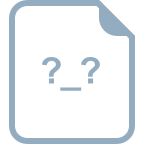














