python利用BiLSTM-CRF在文本数据中提取中文景点实体
时间: 2024-01-21 09:17:23 浏览: 52
BiLSTM-CRF模型是一种常用于命名实体识别的深度学习模型。在中文景点实体提取任务中,可以使用BiLSTM-CRF模型来对文本进行分词、词性标注和实体识别。
以下是一个简单的代码示例,演示如何使用BiLSTM-CRF模型在中文文本中提取景点实体:
```python
import tensorflow as tf
from keras.models import Model, Input
from keras.layers import LSTM, Embedding, Dense, TimeDistributed, Dropout, Bidirectional
from keras_contrib.layers import CRF
# 定义模型参数
EMBED_DIM = 200 # 词向量维度
BiRNN_UNITS = 200 # BiLSTM隐藏层维度
MAX_LEN = 100 # 序列最大长度
VOCAB_SIZE = len(word2id) # 词汇表大小
TAGS_NUM = len(tag2id) # 标签数量
# 定义模型输入
input_layer = Input(shape=(MAX_LEN,))
embedding_layer = Embedding(input_dim=VOCAB_SIZE, output_dim=EMBED_DIM, input_length=MAX_LEN)(input_layer)
dropout_layer = Dropout(0.5)(embedding_layer)
bidirectional_layer = Bidirectional(LSTM(BiRNN_UNITS//2, return_sequences=True))(dropout_layer)
output_layer = TimeDistributed(Dense(TAGS_NUM, activation="softmax"))(bidirectional_layer)
crf_layer = CRF(TAGS_NUM)
output_layer = crf_layer(output_layer)
# 定义模型
model = Model(input_layer, output_layer)
# 编译模型
model.compile(optimizer="adam", loss=crf_layer.loss_function, metrics=[crf_layer.accuracy])
# 训练模型
model.fit(X_train, np.array(y_train), batch_size=32, epochs=10, validation_data=(X_test, np.array(y_test)))
```
在上述代码中,我们使用了Keras和TensorFlow框架来定义和训练BiLSTM-CRF模型。首先,我们定义了模型的输入层,然后通过嵌入层将输入转换为词向量。接着,我们添加了一个Dropout层来减少过拟合,并使用双向LSTM对文本进行编码。最后,我们使用TimeDistributed层将LSTM输出映射到标签空间,并使用CRF层来计算标签序列的概率。我们使用Adam优化器和CRF层的损失函数来编译模型,并在训练集上训练模型。
需要注意的是,上述代码中的X_train和y_train是训练数据的特征矩阵和标签矩阵,分别表示输入的文本序列和实体标签序列。在训练之前,你需要将原始文本数据转换为特征矩阵和标签矩阵。
此外,你还需要定义word2id和tag2id字典,用于将文本和标签转换为数字表示。这些字典可以通过预处理训练数据来获取。
最后,一旦训练好模型,你就可以使用它来对新的文本数据进行实体识别。具体的步骤包括将文本转换为特征矩阵,然后使用模型对特征矩阵进行预测,最后将预测结果转换为标签序列。
相关推荐
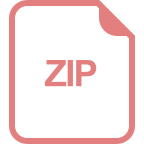
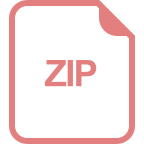
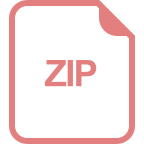
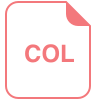
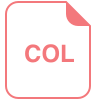
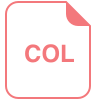
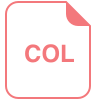









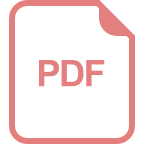
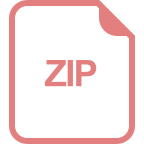