前端点击按钮把数据库中的数据写成多个excel文件导出到本地
时间: 2023-09-30 16:09:41 浏览: 60
要实现这个功能,你需要使用前端框架(如Vue、React等)和后端技术(如Node.js、PHP等)来完成。下面是一个基本的实现步骤:
1. 在前端页面中创建一个按钮,当用户点击该按钮时,向后端发送请求,请求数据。
2. 后端接收到请求后,从数据库中获取数据,并将数据按照一定格式(如JSON)返回给前端。
3. 前端接收到数据后,将数据转换为Excel文件格式。这里可以使用第三方库(如SheetJS)来帮助实现。
4. 将生成的Excel文件保存到本地。这可以通过使用浏览器的下载功能来实现。
下面是一个大致的代码实现示例:
前端代码:
```html
<template>
<div>
<button @click="exportData">导出数据</button>
</div>
</template>
<script>
import axios from 'axios'
import XLSX from 'xlsx'
export default {
methods: {
async exportData() {
const response = await axios.get('/api/data')
const data = response.data
const wb = XLSX.utils.book_new()
data.forEach((item, index) => {
const ws = XLSX.utils.json_to_sheet(item)
XLSX.utils.book_append_sheet(wb, ws, `Sheet ${index + 1}`)
})
XLSX.writeFile(wb, 'data.xlsx')
}
}
}
</script>
```
后端代码(使用Node.js和Express框架):
```javascript
const express = require('express')
const XLSX = require('xlsx')
const { Pool } = require('pg')
const pool = new Pool({
user: 'your_username',
host: 'your_database_host',
database: 'your_database_name',
password: 'your_database_password',
port: 5432
})
const app = express()
app.get('/api/data', async (req, res) => {
const client = await pool.connect()
try {
const result = await client.query('SELECT * FROM your_table')
const data = result.rows
res.json(data)
} finally {
client.release()
}
})
app.listen(3000, () => console.log('Server started on port 3000'))
```
需要注意的是,这只是一个基本的实现示例,你还需要根据自己的具体业务需求进行相应的修改和扩展。
相关推荐
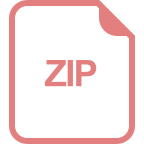
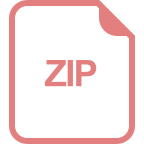
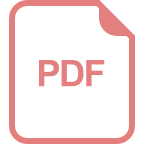














