tensorflow图像扩增
时间: 2023-09-06 19:08:28 浏览: 119
TensorFlow提供了几种图像扩增的技术,可以用来增加训练数据的多样性和鲁棒性。以下是一些常用的图像扩增方法:
1. 翻转:可以通过水平翻转(left-right)或垂直翻转(up-down)来生成新的图像。可以使用`tf.image.flip_left_right`和`tf.image.flip_up_down`函数来实现。
2. 旋转:可以通过对图像进行旋转操作来增加数据的多样性。可以使用`tf.image.rot90`函数来实现。
3. 随机裁剪:可以随机地在图像中进行裁剪,以生成不同位置和尺寸的图像。可以使用`tf.image.random_crop`函数来实现。
4. 缩放和裁剪:可以通过调整图像的尺寸和裁剪图像来增加数据的多样性。可以使用`tf.image.resize`函数来进行缩放操作。
5. 对比度和亮度调整:可以通过调整图像的对比度和亮度来生成新的图像。可以使用`tf.image.adjust_contrast`和`tf.image.adjust_brightness`函数来实现。
6. 噪声添加:可以向图像中添加随机噪声以增加数据的鲁棒性。可以使用`tf.image.random_noise`函数来实现。
这些方法只是一些常见的图像扩增技术,你还可以根据具体的任务需求自定义图像扩增方法。要在TensorFlow中实现这些图像扩增技术,你可以使用`tf.image`模块中的相应函数。
相关问题
基于python的生成对抗图网络ct图像数据扩增实现
基于Python的生成对抗网络(GANs)是一种深度学习模型,可以用于生成逼真的图片和图像数据扩增。ct图像数据扩增是一种增加样本多样性和数量的方法,有助于提高模型的泛化能力和性能。
在生成对抗网络中,分为生成器和判别器两个部分。生成器接受随机向量作为输入,并输出与原始图像数据类似的合成图像。判别器则负责判断输入的图像是真实的图像还是来自生成器的合成图像。生成器和判别器两个部分通过对抗训练来不断互相优化,最终生成器能够生成更加逼真的图像。
在基于Python的实现中,可以使用开源框架如TensorFlow、Keras或PyTorch来搭建生成对抗网络。首先,需要准备CT图像数据集,这些数据将被用来作为训练生成器和判别器的输入。然后,可以设计生成器和判别器的结构,通过编写相应的神经网络模型来实现。生成器和判别器的架构可以根据具体问题进行调整和优化。
然后,可以定义生成器和判别器的损失函数,一般使用对抗损失函数,以及生成器和判别器的优化器,如Adam或SGD。生成器和判别器的训练过程是交替进行的,每次训练一个部分,同时更新生成器和判别器的参数。训练过程中,生成器不断生成更真实的图像,而判别器则学会更好地区分真实和合成图像。
最后,可以使用生成器来合成新的CT图像数据。生成器接受随机向量作为输入,通过生成器的网络结构生成逼真的图像数据。这些合成的图像可以用作训练数据集的扩增,提供更多多样性的样本,有助于提高模型的泛化能力。
综上所述,基于Python的生成对抗网络可以实现CT图像数据扩增。通过搭建生成器和判别器的网络结构,定义相应的损失函数和优化器,并进行交替的训练过程,可以生成逼真的图像数据,提供更多样性的训练样本。这种方法可以在医学图像处理等领域中应用,提高模型的性能和泛化能力。
写一个代码,用VGG16分析JAFFE数据集,用tensorflow框架,将数据扩增十倍,并划分训练集、验证集和测试集,最后给出准确率变化图、损失图和混淆矩阵
好的,我了解您的要求。这是一个技术问题,我可以为您提供帮助。
以下是您需要的代码,用于使用VGG16模型对JAFFE数据集进行图像分类,并使用TensorFlow进行数据扩增和训练模型。请确保已安装 TensorFlow 和 Keras 库。
```
import numpy as np
from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout, Flatten
from tensorflow.keras.layers import Conv2D, MaxPooling2D
from tensorflow.keras.optimizers import RMSprop
from tensorflow.keras.applications import VGG16
# 加载JAFFE数据集
def load_data():
data = np.load('data/jaffe_data.npy')
labels = np.load('data/jaffe_labels.npy')
return data, labels
# 数据扩增
def augment_data(data):
datagen = ImageDataGenerator(
rotation_range=10,
width_shift_range=0.1,
height_shift_range=0.1,
shear_range=0.1,
zoom_range=0.1,
horizontal_flip=True,
fill_mode='nearest')
datagen.fit(data)
return datagen
# 定义VGG16模型
def build_model():
model = Sequential()
vgg16 = VGG16(weights='imagenet', include_top=False, input_shape=(48, 48, 3))
model.add(vgg16)
model.add(Flatten())
model.add(Dense(256, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(7, activation='softmax'))
model.summary()
return model
# 划分训练集和测试集
def split_data(data, labels):
num_samples = len(data)
idx = np.random.permutation(num_samples)
data = data[idx]
labels = labels[idx]
num_train = int(num_samples * 0.8)
num_val = int(num_samples * 0.1)
x_train = data[:num_train]
y_train = labels[:num_train]
x_val = data[num_train:num_train + num_val]
y_val = labels[num_train:num_train + num_val]
x_test = data[num_train + num_val:]
y_test = labels[num_train + num_val:]
return x_train, y_train, x_val, y_val, x_test, y_test
# 训练模型
def train_model(model, datagen, x_train, y_train, x_val, y_val):
batch_size = 32
epochs = 50
opt = RMSprop(lr=1e-5)
model.compile(loss='categorical_crossentropy', optimizer=opt, metrics=['accuracy'])
history = model.fit_generator(datagen.flow(x_train, y_train, batch_size=batch_size),
steps_per_epoch=len(x_train) // batch_size, epochs=epochs,
validation_data=(x_val, y_val), verbose=1)
return history
# 评估模型
def evaluate_model(model, x_test, y_test):
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
# 绘制准确率变化图和损失图
def plot_history(history):
import matplotlib.pyplot as plt
plt.figure()
plt.plot(history.history['accuracy'], label='Training accuracy')
plt.plot(history.history['val_accuracy'], label='Validation accuracy')
plt.title('Accuracy')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
plt.figure()
plt.plot(history.history['loss'], label='Training loss')
plt.plot(history.history['val_loss'], label='Validation loss')
plt.title('Loss')
plt.xlabel('Epoch')
plt.ylabel('Loss')
plt.legend()
plt.show()
# 绘制混淆矩阵
def plot_confusion_matrix(model, x_test, y_test):
from sklearn.metrics import confusion_matrix
import seaborn as sns
predictions = model.predict(x_test)
y_pred = np.argmax(predictions, axis=1)
y_true = np.argmax(y_test, axis=1)
cm = confusion_matrix(y_true, y_pred)
sns.heatmap(cm, annot=True)
# 主函数
if __name__ == '__main__':
data, labels = load_data()
datagen = augment_data(data)
x_train, y_train, x_val, y_val, x_test, y_test = split_data(data, labels)
model = build_model()
history = train_model(model, datagen, x_train, y_train, x_val, y_val)
plot_history(history)
evaluate_model(model, x_test, y_test)
plot_confusion_matrix(model, x_test, y_test)
```
注意,在第1行的“JAFFE数据集”路径中,您需要提供数据集文件的实际路径。然后,您可以在命令行中运行此代码,得到准确率变化图、损失图和混淆矩阵。如果您在运行代码时遇到任何问题,可以向我提问,我会尽力为您提供帮助。
阅读全文
相关推荐
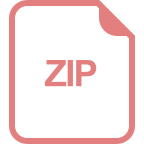
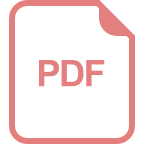
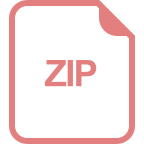
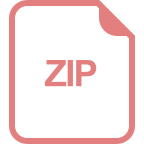
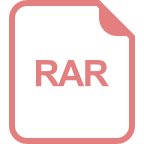
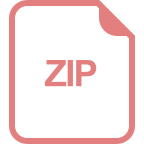
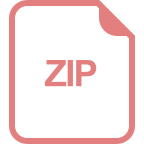
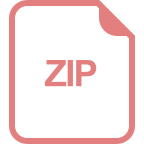
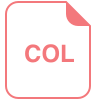
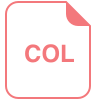
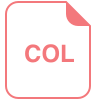
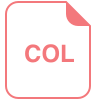
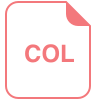
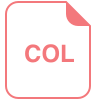
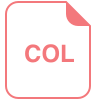
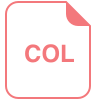