c/c++截取字符串string
时间: 2023-07-24 09:35:57 浏览: 57
在 C++ 中,可以使用 `substr` 函数来截取一个 `string` 类型的字符串。
`substr` 函数的语法如下:
```c++
string substr (size_t pos, size_t len) const;
```
其中,`pos` 是起始位置,`len` 是截取的长度。
例如,要截取一个 `string` 类型的字符串 `str` 的前 5 个字符,可以使用以下代码:
```c++
string str = "Hello, world!";
string result = str.substr(0, 5);
```
上述代码会将字符串 `str` 中从位置 0 开始的 5 个字符截取出来,并赋值给 `result`。
需要注意的是,`substr` 函数会返回一个新的 `string` 对象,因此原有的 `string` 对象不会受到影响。
相关问题
c++ 搜索//字符串截取字符串
回文数是指正着读和反着读都一样的数字。在C/C++中,可以使用数组和字符串来判断一个数是否为回文数。
使用数组的方法是将数字的每一位存储到数组中,然后从两端开始比较,如果相同则继续比较,直到中间位置。如果所有位都相同,则该数为回文数。
使用字符串的方法是将数字转换为字符串,然后从两端开始比较,如果相同则继续比较,直到中间位置。如果所有字符都相同,则该数为回文数。
下面是使用数组的示例代码:
```c++
#include <iostream>
using namespace std;
bool isPalindrome(int num) {
int digits[10];
int i = 0;
while (num > 0) {
digits[i++] = num % 10;
num /= 10;
}
for (int j = 0; j < i / 2; j++) {
if (digits[j] != digits[i - j - 1]) {
return false;
}
}
return true;
}
int main() {
int num;
cout << "请输入一个数字:";
cin >> num;
if (isPalindrome(num)) {
cout << num << " 是回文数" << endl;
} else {
cout << num << " 不是回文数" << endl;
}
return 0;
}
```
下面是使用字符串的示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
bool isPalindrome(int num) {
string str = to_string(num);
int len = str.length();
for (int i = 0; i < len / 2; i++) {
if (str[i] != str[len - i - 1]) {
return false;
}
}
return true;
}
int main() {
int num;
cout << "请输入一个数字:";
cin >> num;
if (isPalindrome(num)) {
cout << num << " 是回文数" << endl;
} else {
cout << num << " 不是回文数" << endl;
}
return 0;
}
```
c++string截取字符串
### 回答1:
你可以使用 `substr` 函数来截取 C++ 中的字符串。该函数的语法如下:
```c++
string substr (size_t pos, size_t len) const;
```
其中,`pos` 是从哪个位置开始截取,`len` 是截取的长度。例如,要截取字符串 `str` 的第 3 个字符开始的 5 个字符,可以这样写:
```c++
string str = "hello world";
string substr = str.substr(2, 5); // 从第3个字符开始,截取5个字符
cout << substr << endl; // 输出:lo wo
```
需要注意,`substr` 函数返回的是截取后的子字符串,原字符串不会受到影响。
### 回答2:
在C语言中,可以使用多种方法来截取字符串。其中一种常见的方法是使用函数库中的字符串处理函数来实现。
其中,可以使用"strncpy"函数来截取字符串。该函数的原型为:
```
char* strncpy(char* destination, const char* source, size_t num);
```
参数说明:
- destination:目标字符串的指针,用于存储截取后的结果;
- source:源字符串的指针,即需要被截取的字符串;
- num:要截取的字符个数。
使用方法如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[10];
strncpy(destination, source, 5);
destination[5] = '\0';
printf("截取后的字符串是:%s\n", destination);
return 0;
}
```
以上代码将会输出:"截取后的字符串是:Hello"。
需要注意的是,在截取字符串时,需要留出足够的空间存储截取后的结果,并且手动添加字符串结束符"\0"。
除了使用"strncpy"函数外,还可以使用其他的字符串处理函数,如"strtok"、"strstr"等,根据具体需求选择合适的函数来截取字符串。
### 回答3:
在C++中,可以使用一些方法来截取字符串。具体步骤如下:
1. 使用C++的字符串类来存储和操作字符串。可以使用`std::string`来代替C语言中的字符数组。
2. 使用`substr()`函数来实现字符串的截取。`substr()`函数有两个参数,第一个参数是截取的开始位置,从0开始计数,第二个参数是截取的长度。
3. 通过指定开始位置和截取长度,可以截取想要的子字符串。例如,如果要截取从位置2开始的长度为5的子字符串,可以使用`substr(2, 5)`来实现。
下面是一个简单的例子来演示如何在C++中截取字符串:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string subStr = str.substr(2, 5);
std::cout << "截取的子字符串为: " << subStr << std::endl;
return 0;
}
```
上述例子中,原始字符串为"Hello, World!",使用`substr()`函数截取的开始位置为2,长度为5的子字符串。输出结果为:"截取的子字符串为: llo, "。
以上就是在C++中使用C字符串截取字符串的基本方法。希望对你有帮助!
相关推荐
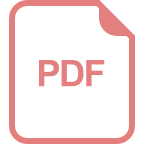
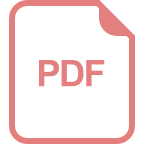
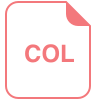
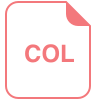
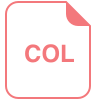
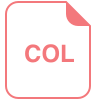
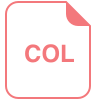







