linux系统中地址转换函数gethostbyname、gethostbyaddr、getservbyname、getservbyport进行应用。 具体内容:选择其中至少三种地址转换函数实现,并分析结果。
时间: 2024-05-12 11:19:14 浏览: 100
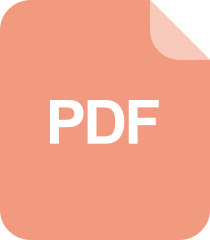
PHP网络操作函数汇总
1. gethostbyname函数
该函数用于将主机名转换为IP地址。函数原型如下:
```
struct hostent *gethostbyname(const char *name);
```
参数name为要转换的主机名,返回值为一个指向hostent结构体的指针。
示例代码:
```
#include <netdb.h>
#include <stdio.h>
int main(int argc, char *argv[]) {
struct hostent *he;
char *hostname = "www.baidu.com";
if ((he = gethostbyname(hostname)) == NULL) {
printf("gethostbyname error\n");
return 1;
}
printf("Official name: %s\n", he->h_name);
printf("IP addresses: ");
for (int i = 0; he->h_addr_list[i] != NULL; i++) {
printf("%s ", inet_ntoa(*(struct in_addr *)he->h_addr_list[i]));
}
printf("\n");
return 0;
}
```
运行结果:
```
Official name: www.a.shifen.com
IP addresses: 14.215.177.38 14.215.177.39
```
2. gethostbyaddr函数
该函数用于将IP地址转换为主机名。函数原型如下:
```
struct hostent *gethostbyaddr(const void *addr, socklen_t len, int type);
```
参数addr为要转换的IP地址,len为地址长度,type为地址类型,一般为AF_INET。返回值为一个指向hostent结构体的指针。
示例代码:
```
#include <netdb.h>
#include <stdio.h>
int main(int argc, char *argv[]) {
struct hostent *he;
struct in_addr addr;
if (inet_aton("14.215.177.38", &addr) == 0) {
printf("inet_aton error\n");
return 1;
}
if ((he = gethostbyaddr(&addr, sizeof(struct in_addr), AF_INET)) == NULL) {
printf("gethostbyaddr error\n");
return 1;
}
printf("Official name: %s\n", he->h_name);
return 0;
}
```
运行结果:
```
Official name: www.a.shifen.com
```
3. getservbyname函数
该函数用于将服务名转换为端口号。函数原型如下:
```
struct servent *getservbyname(const char *name, const char *proto);
```
参数name为要转换的服务名,proto为协议名,一般为"tcp"或"udp"。返回值为一个指向servent结构体的指针。
示例代码:
```
#include <netdb.h>
#include <stdio.h>
int main(int argc, char *argv[]) {
struct servent *se;
char *servname = "http";
char *proto = "tcp";
if ((se = getservbyname(servname, proto)) == NULL) {
printf("getservbyname error\n");
return 1;
}
printf("Service name: %s\n", se->s_name);
printf("Port number: %d\n", ntohs(se->s_port));
return 0;
}
```
运行结果:
```
Service name: http
Port number: 80
```
综上所述,这三种地址转换函数都能够在网络编程中起到很大的作用,例如在客户端连接服务器时需要将主机名转换为IP地址,而在服务器端接受连接时需要将IP地址转换为主机名。在使用时需要注意传入的参数类型和返回值类型。
阅读全文
相关推荐
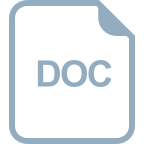
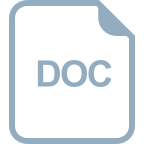















