使用react写一个滚动抽奖的代码
时间: 2024-03-21 16:38:15 浏览: 24
好的,以下是一个简单的React滚动抽奖组件的代码示例:
```jsx
import React, { useState } from "react";
import axios from "axios";
const Lottery = () => {
const [prizes, setPrizes] = useState([]);
const [rolling, setRolling] = useState(false);
const [result, setResult] = useState(null);
const startRolling = () => {
setRolling(true);
// 假设抽奖后端接口返回的数据格式为:{id: 1, name: '奖品1'}
axios.get("/api/lottery").then((response) => {
setPrizes(response.data);
const randomIndex = Math.floor(Math.random() * response.data.length);
setResult(response.data[randomIndex]);
setRolling(false);
});
};
return (
<div>
<div className="lottery-container">
{rolling ? (
<div className="rolling">
<div className="rolling-item">奖品1</div>
<div className="rolling-item">奖品2</div>
<div className="rolling-item">奖品3</div>
<div className="rolling-item">奖品4</div>
<div className="rolling-item">奖品5</div>
<div className="rolling-item">奖品6</div>
<div className="rolling-item">奖品7</div>
<div className="rolling-item">奖品8</div>
<div className="rolling-item">奖品9</div>
<div className="rolling-item">奖品10</div>
</div>
) : (
<div className="lottery-result">{result ? result.name : ""}</div>
)}
</div>
<button onClick={startRolling} disabled={rolling}>
{rolling ? "抽奖中..." : "开始抽奖"}
</button>
</div>
);
};
export default Lottery;
```
这个组件包含一个抽奖区域和一个抽奖按钮。当用户点击抽奖按钮时,组件会向后端发送请求以获取奖品数据,并开始滚动抽奖区域。当抽奖结束时,组件会显示抽奖结果。
相关推荐
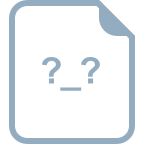
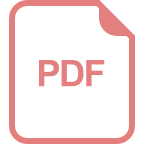














