1. 用户基本信息自定义功能:用户可以自行设定自己的基本信息,如用户名、性别、年龄、联系方式等。Javaweb代码
时间: 2023-06-27 20:04:36 浏览: 48
以下是一个简单的用户基本信息自定义功能的Javaweb代码示例:
1. 创建数据库表
在数据库中创建一个名为"user_info"的表,包含以下列:
- id (int, primary key, auto_increment)
- username (varchar(50), not null)
- gender (varchar(10))
- age (int)
- contact (varchar(50))
2. 创建JavaBean类
创建一个名为"UserInfo"的JavaBean类,包含以下属性和方法:
```java
public class UserInfo {
private int id;
private String username;
private String gender;
private int age;
private String contact;
// getter and setter methods
public int getId() { return id; }
public void setId(int id) { this.id = id; }
public String getUsername() { return username; }
public void setUsername(String username) { this.username = username; }
public String getGender() { return gender; }
public void setGender(String gender) { this.gender = gender; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
public String getContact() { return contact; }
public void setContact(String contact) { this.contact = contact; }
}
```
3. 创建DAO类
创建一个名为"UserInfoDAO"的DAO类,实现用户基本信息的增删改查功能。以下是一个简单的示例:
```java
public class UserInfoDAO {
// 数据库连接配置
private static final String DB_URL = "jdbc:mysql://localhost:3306/test";
private static final String DB_USERNAME = "root";
private static final String DB_PASSWORD = "root";
// 插入用户信息
public void insert(UserInfo userInfo) throws SQLException {
Connection conn = null;
PreparedStatement ps = null;
try {
// 获取数据库连接
conn = DriverManager.getConnection(DB_URL, DB_USERNAME, DB_PASSWORD);
// 构造SQL语句
String sql = "INSERT INTO user_info (username, gender, age, contact) VALUES (?, ?, ?, ?)";
ps = conn.prepareStatement(sql);
// 设置SQL参数
ps.setString(1, userInfo.getUsername());
ps.setString(2, userInfo.getGender());
ps.setInt(3, userInfo.getAge());
ps.setString(4, userInfo.getContact());
// 执行SQL语句
ps.executeUpdate();
} finally {
// 关闭数据库连接和PreparedStatement对象
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
}
// 更新用户信息
public void update(UserInfo userInfo) throws SQLException {
Connection conn = null;
PreparedStatement ps = null;
try {
// 获取数据库连接
conn = DriverManager.getConnection(DB_URL, DB_USERNAME, DB_PASSWORD);
// 构造SQL语句
String sql = "UPDATE user_info SET username=?, gender=?, age=?, contact=? WHERE id=?";
ps = conn.prepareStatement(sql);
// 设置SQL参数
ps.setString(1, userInfo.getUsername());
ps.setString(2, userInfo.getGender());
ps.setInt(3, userInfo.getAge());
ps.setString(4, userInfo.getContact());
ps.setInt(5, userInfo.getId());
// 执行SQL语句
ps.executeUpdate();
} finally {
// 关闭数据库连接和PreparedStatement对象
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
}
// 删除用户信息
public void delete(int id) throws SQLException {
Connection conn = null;
PreparedStatement ps = null;
try {
// 获取数据库连接
conn = DriverManager.getConnection(DB_URL, DB_USERNAME, DB_PASSWORD);
// 构造SQL语句
String sql = "DELETE FROM user_info WHERE id=?";
ps = conn.prepareStatement(sql);
// 设置SQL参数
ps.setInt(1, id);
// 执行SQL语句
ps.executeUpdate();
} finally {
// 关闭数据库连接和PreparedStatement对象
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
}
// 查询用户信息
public UserInfo select(int id) throws SQLException {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
UserInfo userInfo = null;
try {
// 获取数据库连接
conn = DriverManager.getConnection(DB_URL, DB_USERNAME, DB_PASSWORD);
// 构造SQL语句
String sql = "SELECT * FROM user_info WHERE id=?";
ps = conn.prepareStatement(sql);
// 设置SQL参数
ps.setInt(1, id);
// 执行SQL语句
rs = ps.executeQuery();
// 处理查询结果
if (rs.next()) {
userInfo = new UserInfo();
userInfo.setId(rs.getInt("id"));
userInfo.setUsername(rs.getString("username"));
userInfo.setGender(rs.getString("gender"));
userInfo.setAge(rs.getInt("age"));
userInfo.setContact(rs.getString("contact"));
}
} finally {
// 关闭ResultSet、PreparedStatement和数据库连接
if (rs != null) {
rs.close();
}
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
return userInfo;
}
}
```
4. 创建Servlet类
创建一个名为"UserInfoServlet"的Servlet类,处理用户提交的基本信息表单,并调用DAO类实现数据的增删改查功能。以下是一个简单的示例:
```java
public class UserInfoServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private UserInfoDAO userInfoDAO = new UserInfoDAO();
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 处理GET请求,查询用户信息并返回到页面
String idStr = request.getParameter("id");
if (idStr != null && !idStr.isEmpty()) {
int id = Integer.parseInt(idStr);
UserInfo userInfo = null;
try {
userInfo = userInfoDAO.select(id);
} catch (SQLException e) {
e.printStackTrace();
}
request.setAttribute("userInfo", userInfo);
}
request.getRequestDispatcher("userinfo.jsp").forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 处理POST请求,保存用户信息并返回到页面
String idStr = request.getParameter("id");
String username = request.getParameter("username");
String gender = request.getParameter("gender");
String ageStr = request.getParameter("age");
String contact = request.getParameter("contact");
UserInfo userInfo = new UserInfo();
if (idStr != null && !idStr.isEmpty()) {
// 更新用户信息
int id = Integer.parseInt(idStr);
userInfo.setId(id);
}
userInfo.setUsername(username);
userInfo.setGender(gender);
if (ageStr != null && !ageStr.isEmpty()) {
int age = Integer.parseInt(ageStr);
userInfo.setAge(age);
}
userInfo.setContact(contact);
try {
if (userInfo.getId() == 0) {
// 插入新用户信息
userInfoDAO.insert(userInfo);
} else {
// 更新用户信息
userInfoDAO.update(userInfo);
}
} catch (SQLException e) {
e.printStackTrace();
}
request.setAttribute("userInfo", userInfo);
request.getRequestDispatcher("userinfo.jsp").forward(request, response);
}
}
```
5. 创建JSP页面
创建一个名为"userinfo.jsp"的JSP页面,包含一个基本信息表单,用于提交和显示用户信息。以下是一个简单的示例:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>User Info</title>
</head>
<body>
<h1>User Info</h1>
<form method="post" action="userinfo">
<input type="hidden" name="id" value="${userInfo.id}">
<p>
<label>Username:</label>
<input type="text" name="username" value="${userInfo.username}">
</p>
<p>
<label>Gender:</label>
<input type="radio" name="gender" value="male" ${userInfo.gender == 'male' ? 'checked' : ''}>Male
<input type="radio" name="gender" value="female" ${userInfo.gender == 'female' ? 'checked' : ''}>Female
</p>
<p>
<label>Age:</label>
<input type="number" name="age" value="${userInfo.age}">
</p>
<p>
<label>Contact:</label>
<input type="text" name="contact" value="${userInfo.contact}">
</p>
<p>
<input type="submit" value="Save">
</p>
</form>
</body>
</html>
```
6. 部署和运行
将所有代码打包成一个WAR文件,部署到Tomcat服务器上,启动Tomcat服务器,在浏览器中访问"http://localhost:8080/userinfo",即可看到用户基本信息表单。用户可以填写、修改自己的基本信息,并保存到数据库中。在浏览器中访问"http://localhost:8080/userinfo?id=1",可以查询指定用户的基本信息。
相关推荐
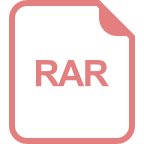
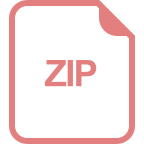
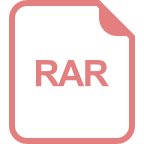















