根据上述步骤,编写代码
时间: 2024-10-13 09:07:31 浏览: 25
根据上述步骤,下面是一个简化的C++示例代码片段,展示如何进行基本的点云粗解和颜色赋值。请注意,这只是一个基础的示例,实际项目中可能需要更复杂的错误处理和优化:
```cpp
#include <opencv2/opencv.hpp>
#include <fstream>
// 假设我们已经得到了两幅图像的特征点和描述符
cv::Mat imgA_descriptors, imgB_descriptors;
std::vector<cv::Point2f> imgA_keypoints, imgB_keypoints;
// 使用某种匹配器进行匹配(这里假设使用BruteForceMatcher)
cv::BFMatcher matcher(NORM_L2);
std::vector<std::DMatch> matches;
matcher.match(imgA_descriptors, imgB_descriptors, matches);
// 对匹配进行筛选(比如设定阈值)
std::vector<cv::DMatch> goodMatches;
std::copy_if(matches.begin(), matches.end(), std::back_inserter(goodMatches), [](const cv::DMatch& match) { return match.distance <= 0.8 * match.distance.max(); });
// 构建对应矩阵并解算相机位姿
cv::Mat H = findHomography(imgA_keypoints, imgB_keypoints, matches, CV_RANSAC, 3); // 使用RANSAC去除异常匹配
// 从imgB获取对应像素颜色
cv::Mat imgB_color;
std::vector<cv::Point2i> srcPoints, dstPoints;
for (const auto& match : goodMatches)
{
int index = match.queryIdx;
srcPoints.push_back(imgA_keypoints[index]);
dstPoints.push_back(match.trainIdx == -1 ? cv::Point2i() : imgB_keypoints[match.trainIdx]);
}
cv::goodFeaturesToTrack(imgB, imgB_color, srcPoints.size(), 0.01, 10, NO_CONTOURS, dstPoints);
cv::warpPerspective(imgB_color, imgB_color, H, imgB_color.size());
// 将点云转换为txt格式
std::ofstream output("output.txt");
for (size_t i = 0; i < srcPoints.size(); ++i)
{
const float* point = srcPoints[i].pt;
const uint8_t* color = imgB_color.at<cv::Vec3b>(srcPoints[i].y, srcPoints[i].x);
output << point[0] << " " << point[1] << " " << point[2] << " " << color[2] << " " << color[1] << " " << color[0] << "\n";
}
output.close();
```
这段代码展示了如何匹配特征、估计相机姿态、获取像素颜色并保存到文件。实际应用中,你可能需要根据具体需求调整细节,例如选择不同的匹配方法、添加错误检查等。
阅读全文
相关推荐










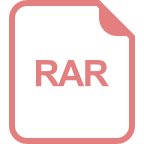


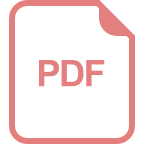




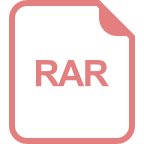