C++里面统计字符个数
时间: 2024-12-14 15:05:20 浏览: 42
在C++中,你可以通过遍历字符串并使用计数器来统计字符个数。下面是一个简单的示例,展示了如何计算字符串中特定字符的数量:
```cpp
#include <iostream>
#include <string>
int countCharacters(const std::string& str, char character) {
int count = 0;
for (char c : str) {
if (c == character) {
count++;
}
}
return count;
}
int main() {
std::string input = "Hello, C++!";
char targetCharacter = 'o';
int occurrence = countCharacters(input, targetCharacter);
std::cout << "The character '" << targetCharacter << "' appears " << occurrence << " times in the string." << std::endl;
return 0;
}
```
在这个例子中,`countCharacters` 函数接受一个字符串 `str` 和一个字符 `character`,然后通过遍历字符串,每次遇到目标字符就增加计数器。最后返回这个字符在字符串中出现的次数。
相关问题
用c++写统计一串字符里面的英文字母
可以使用C语言中的字符数组和循环语句来统计一串字符中的英文字母。具体实现可以参考以下代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int count[26] = {0}; // 用于存储每个英文字母出现的次数
printf("请输入一串字符:");
fgets(str, sizeof(str), stdin);
for (int i = 0; str[i] != '\0'; i++) {
if (isalpha(str[i])) { // 判断是否为英文字母
count[tolower(str[i]) - 'a']++; // 统计出现次数
}
}
printf("英文字母出现次数如下:\n");
for (int i = 0; i < 26; i++) {
if (count[i] > 0) {
printf("%c: %d\n", 'a' + i, count[i]);
}
}
return 0;
}
```
这段代码可以读入一串字符,然后统计其中的英文字母出现次数,并输出结果。
从键盘输入一个字符串,统计里面数字1的个数,数字2的个数和字符a的个数c++
在C++中,你可以使用标准库函数`std::cin`从键盘接收输入,并使用`std::string::count()`函数来统计特定字符的数量。以下是实现这个功能的一个简单示例:
```cpp
#include <iostream>
#include <string>
int main() {
std::string input;
int count1 = 0, count2 = 0, count_a = 0;
// 从键盘读取输入
std::cout << "请输入一个字符串: ";
getline(std::cin, input); // 使用getline避免换行符影响计数
// 遍历字符串并统计1、2和'a'
for (char c : input) {
if (c == '1') {
count1++;
} else if (c == '2') {
count2++;
} else if (c == 'a') {
count_a++;
}
}
// 输出结果
std::cout << "数字1的数量: " << count1 << std::endl;
std::cout << "数字2的数量: " << count2 << std::endl;
std::cout << "字符'a'的数量: " << count_a << std::endl;
return 0;
}
阅读全文
相关推荐





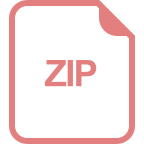

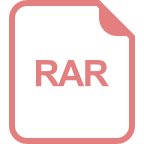








