java使用ftp上传文件到指定服务器
时间: 2024-09-26 18:18:13 浏览: 55
在Java中,使用FTP (File Transfer Protocol) 上传文件到指定服务器通常需要使用第三方库,比如Apache Commons Net 或者 Java自带的JSch等。以下是使用`Apache Commons Net`库的一个基本示例:
```java
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPFile;
public class FTPUploader {
public static void main(String[] args) {
String server = "your_server_address";
int port = 21; // FTP默认端口,也可以设置为其他
String username = "your_username";
String password = "your_password";
FTPClient client = new FTPClient();
try {
// 连接到服务器
client.connect(server, port);
// 登录
client.login(username, password);
// 设置工作目录
client.changeWorkingDirectory("/path/to/remote/directory");
// 上传文件
File fileToUpload = new File("local_file_path");
FileInputStream fis = new FileInputStream(fileToUpload);
boolean uploaded = client.storeFile(fileToUpload.getName(), fis);
if (!uploaded) {
System.out.println("Failed to upload the file.");
} else {
System.out.println("File uploaded successfully.");
}
// 关闭连接
client.logout();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
client.disconnect();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
阅读全文
相关推荐

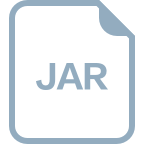














