Visual studio 2019 如何用C#实现打开相机并拍照保存的功能
时间: 2024-09-29 22:10:53 浏览: 102
在 Visual Studio 2019 中使用 C# 实现打开相机并拍照保存的功能,你需要使用 Windows Presentation Foundation (WPF) 的 `System.Windows.Media.Imaging` 和 `Windows.Storage` 模块,以及 `MediaCapture` 类。以下是一个简单的步骤指南:
1. 首先,请确保你的项目引用了以下 NuGet 包(如果尚未安装):
- `Microsoft.NETCore.UniversalWindowsPlatform`
- `WindowsPreview.Kinect`
2. 添加权限:
在 `App.xaml.cs` 文件中,添加对摄像头访问的权限:
```csharp
using Windows.Security.Authentication.Web;
...
protected override async void OnLaunched(LaunchActivatedEventArgs e)
{
// 其他代码...
// 请求摄像头访问权限
var cameraAccess = await MediaCapture.RequestAccessAsync();
if (!cameraAccessgranted)
{
// 处理权限请求失败的情况
}
}
```
3. 创建 `MediaCapture` 对象:
```csharp
private MediaCapture _mediaCapture;
public MainPage()
{
InitializeComponent();
// 初始化媒体捕捉
_mediaCapture = new MediaCapture();
InitializeCamera();
}
private async Task InitializeCamera()
{
try
{
await _mediaCapture.InitializeAsync(new MediaCaptureInitializationSettings
{
StreamingCaptureMode = StreamingCaptureMode.Video,
PhotoCaptureSource = PhotoCaptureSource.Cameras
});
}
catch (Exception ex)
{
// 处理初始化异常
}
}
```
4. 获取照片:
当准备拍摄时,调用 `CapturePhotoToStreamAsync` 方法:
```csharp
private async void TakePictureButton_Click(object sender, RoutedEventArgs e)
{
try
{
// 获取图片流
using (var stream = await _mediaCapture.CreatePhotoBufferAsync())
{
// 使用 SoftwareBitmap 将图像转换为可显示格式
var softwareBitmap = await BitmapEncoder.CreateSoftwareBitmapAsync(stream);
// 将图像保存到文件
await SaveImageToFile(softwareBitmap);
}
}
catch (Exception ex)
{
// 处理拍照或保存异常
}
}
private async Task SaveImageToFile(SoftwareBitmap softwareBitmap)
{
using (var fileStream = await Windows.Storage.ApplicationData.Current.LocalFolder.CreateFileAsync("image.jpg", CreationCollisionOption.ReplaceExisting))
{
var encoder = await BitmapEncoder.CreateJpgEncoderAsync(fileStream);
await encoder.SetSoftwareBitmapAsync(softwareBitmap);
await encoder.FlushAsync();
}
}
```
5. 最后,在关闭应用程序之前别忘了释放 `MediaCapture` 对象资源:
```csharp
protected override void OnSuspending(object sender, SuspendingEventArgs e)
{
base.OnSuspending(e);
// 释放资源
_mediaCapture?.Dispose();
_mediaCapture = null;
// 其他缓存清理操作...
}
```
阅读全文
相关推荐
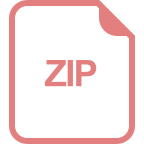
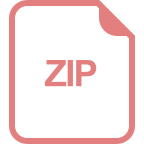
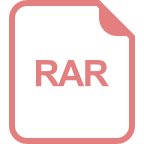
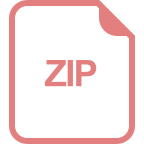
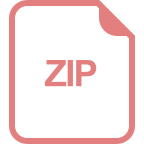
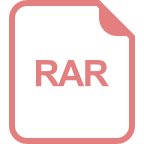
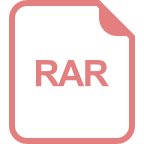
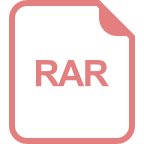
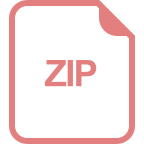
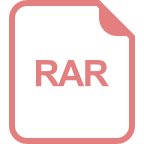
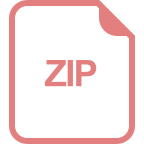
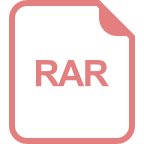
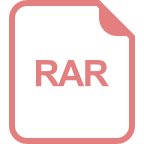
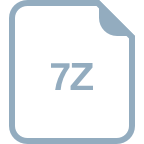
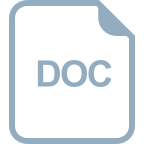
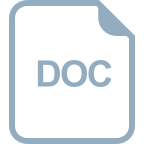
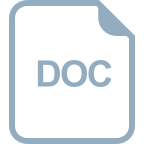
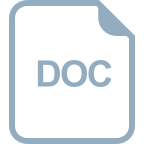
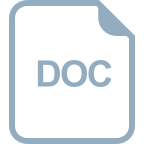
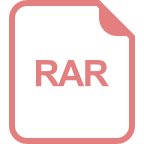