c语言怎么做乘法运算
时间: 2024-09-23 13:03:06 浏览: 96
在C语言中,做乘法运算非常基础,你可以使用`*`操作符来进行。下面是一个简单的例子,展示了如何让两个整数相乘:
```c
#include <stdio.h>
int main() {
int a = 5; // 第一个数
int b = 3; // 第二个数
int result = a * b; // 使用 * 运算符进行乘法
printf("The product of %d and %d is %d.\n", a, b, result);
return 0;
}
```
运行这段程序,会输出 `The product of 5 and 3 is 15.`。
如果你想让变量能接受不同类型的数据(如整型、浮点型),可以将结果类型声明为`double`,并同样使用`*`运算符:
```c
#include <stdio.h>
double multiply(double a, double b) {
return a * b;
}
int main() {
double num1 = 2.5;
double num2 = 4.8;
double product = multiply(num1, num2);
printf("The product of %.1f and %.1f is %.1f.\n", num1, num2, product);
return 0;
}
```
相关问题
c语言做大数运算a*b+c
在C语言中,处理大数运算(如长整型乘法和加法)通常涉及到手动处理溢出问题,因为C标准库并不直接支持无限精度的大数操作。一种常见的方式是通过数组或结构体来存储大数,并利用循环来逐位相乘和相加。
例如,可以创建两个数组(或链表)分别存储两个大数的每一位,然后对应位置进行乘法和加法。这里是一个简单的例子,展示了如何使用数组来实现大数乘法:
```c
#include <stdio.h>
#define MAX_DIGITS 100 // 假设最大数字有100位
typedef struct {
int digits[MAX_DIGITS];
int size; // 数字的位数,不包括前导零
} BigNumber;
// 助记符函数,例如:add_one(bn, carry)
void add_one(BigNumber* bn, int carry) {
if (bn->size == 0 || carry == 0) return;
++(bn->digits[bn->size - 1]);
carry += (bn->digits[bn->size - 1] > 9);
bn->digits[bn->size - 1] %= 10;
if (carry != 0) {
++bn->size;
}
}
// 大数乘法示例,这里只给出了乘法的基本思路,实际应用需要完整处理进位过程
BigNumber multiply(BigNumber a, BigNumber b) {
BigNumber result = {0, 0}; // 初始化结果为0
for (int i = 0; i < a.size; ++i) {
BigNumber temp = {0, 0};
for (int j = 0; j < b.size; ++j) {
temp.digits[temp.size++] = a.digits[i] * b.digits[j]; // 乘法得到当前位的结果
}
add_one(&temp, a.digits[i]); // 向左移位并加上原始的a[i]
add_one(&result, temp.digits[temp.size - 1]); // 累加进位
}
return result;
}
int main() {
// 实例化并初始化两个大数...
BigNumber a, b, result;
// ...然后进行乘法运算...
result = multiply(a, b);
// 输出结果
for (int i = result.size - 1; i >= 0; --i) {
printf("%d", result.digits[i]);
}
return 0;
}
```
注意这只是一个简化版的例子,实际的大数运算需要更复杂的算法来处理溢出和进位情况,以及可能的优化。
完成复数乘法运算。输入两个复数,输出其乘积。(c语言)
在C语言中,我们可以使用结构体和指针来完成复数的乘法运算。首先,我们需要定义一个表示复数的结构体:
```c
typedef struct {
double real; // 实部
double imag; // 虚部
} Complex;
```
接下来,我们创建一个函数来进行复数相乘:
```c
Complex multiplyComplex(Complex c1, Complex c2) {
Complex result;
// 复数乘法公式: (a + bi)(c + di) = (ac - bd) + i(ad + bc)
result.real = c1.real * c2.real - c1.imag * c2.imag;
result.imag = c1.real * c2.imag + c1.imag * c2.real;
return result;
}
```
在这个函数中,`c1` 和 `c2` 分别代表两个输入的复数,返回值是一个新的复数,表示它们的乘积。
如果需要从用户那里获取输入并调用这个函数,可以这样做:
```c
void inputAndPrint() {
printf("请输入第一个复数的实部和虚部,用空格分隔:");
double a, b;
scanf("%lf %lf", &a, &b);
Complex c1 = {a, b};
printf("请输入第二个复数的实部和虚部,用空格分隔:");
double c, d;
scanf("%lf %lf", &c, &d);
Complex c2 = {c, d};
Complex product = multiplyComplex(c1, c2);
printf("两复数相乘的结果是: (%lf, %lf)\n", product.real, product.imag);
}
int main() {
inputAndPrint();
return 0;
}
```
当你运行这个程序时,它会提示你输入两个复数,然后计算并显示它们的乘积。
阅读全文
相关推荐
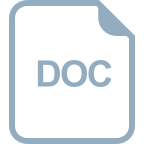
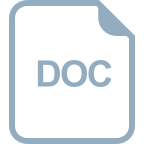
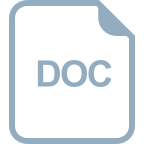
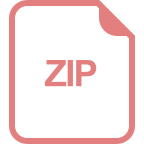
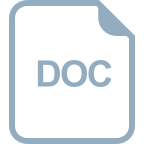
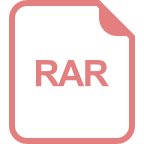
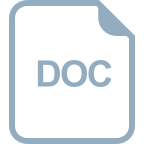
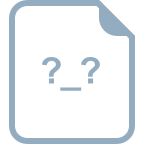
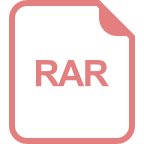
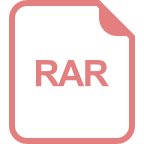
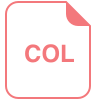
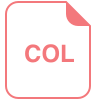




