给了源数据怎么用python雷达图
时间: 2024-10-12 20:12:43 浏览: 24
给定源数据想要制作Python的雷达图,通常你需要一个包含各个维度数据的DataFrame或列表结构。首先,我们需要安装一些绘图库,如`matplotlib`和`seaborn`。以下是一个基本步骤:
1. **准备数据**:数据应该是一个二维数组或Pandas DataFrame,其中每一行代表一个观测点,每一列代表一个特征。
```python
import pandas as pd
# 示例数据
data = {
'特征1': [1, 2, 3],
'特征2': [4, 5, 6],
'特征3': [7, 8, 9]
}
df = pd.DataFrame(data)
```
2. **安装依赖**:
```bash
pip install matplotlib seaborn
```
3. **绘制雷达图**:
```python
from matplotlib import cm
import matplotlib.pyplot as plt
import numpy as np
plt.style.use('_mpl-gallery-nogrid')
def radar_factory(num_vars, frame='circle'):
"""Create a RadarAxes instance with `num_vars` axes."""
# Create circle for polar plots
theta = np.linspace(0, 2 * np.pi, num_vars, endpoint=False)
def draw_circle(ax):
ax.set_xlim(-1, 1)
ax.set_xticks([])
ax.set_ylim(-1, 1)
ax.set_yticks([])
ax.plot(theta, np.ones(num_vars), color="black", linewidth=1)
ax.plot(theta, -np.ones(num_vars), color="black", linewidth=1)
ax.fill(theta, 1, color="#EBF5FB", alpha=0.2)
ax.fill(theta, -1, color="#EBF5FB", alpha=0.2)
if frame == 'polygon':
ax = plt.axes(projection='polar', facecolor="None")
ax.set_thetagrids(theta * 180 / np.pi, labels=data.columns)
draw_circle(ax)
elif frame == 'circle':
ax = plt.subplot(num_vars, 1, 1, projection='polar')
ax.set_rgrids([0.2, 0.4, 0.6, 0.8], angle=theta * 180 / np.pi)
ax.set_axisbelow(True)
draw_circle(ax)
else:
raise ValueError("unknown value for `frame`: %s" % frame)
return ax
ax = radar_factory(df.shape[1])
ax.set_title('雷达图示例')
# 将DataFrame的值映射到颜色
cmap = cm.get_cmap('viridis', 3)
colors = cmap(np.arange(len(df)))
for i, row in df.iterrows():
angles = row.index.values
values = row.values.flatten()
angles += np.pi / 2 # put the first point at the top
angles *= -1 # clockwise rotation
ax.plot(angles, values, linewidth=1, linestyle='solid', c=colors[i])
plt.show()
```
阅读全文
相关推荐
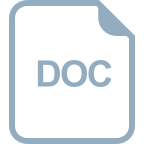
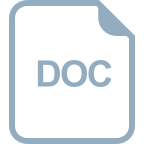
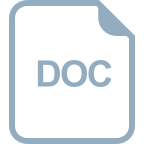
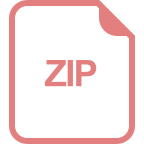
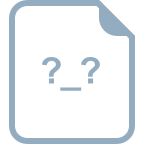










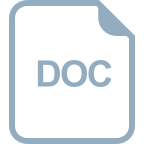
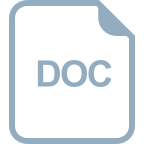