用C语言编写函数,删除整型数组a中值为x的所有元素。给数组赋值时,可以以字符串的形式输入,然后将各个字符转换成数字,从头开始对数组元素逐个进行比较,遇到x就将后面的元素前移一个位置,可删除所有的x。
时间: 2024-12-20 13:22:52 浏览: 7
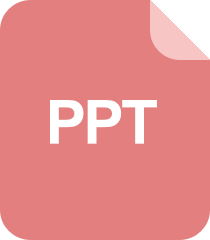
精品课件资料用C语言编写程序.ppt
在C语言中,你可以创建一个函数来实现这个功能。下面是一个简单的示例,我们首先会将输入的字符串分割成整数数组,然后遍历数组,如果找到值为x的元素,就移动后面的元素到前面,并减少数组长度:
```c
#include <stdio.h>
#include <string.h>
// 函数声明
void removeX(int* arr, int size, int x);
// 将字符串转换为整数数组
int* stringToIntArray(char* str, int* count) {
char* end;
*count = 0;
while ((end = strchr(str, ',')) != NULL) {
*count += 1;
str = end + 1;
}
*count++; // 最后一个元素不需要逗号
int* array = (int*)malloc(*count * sizeof(int));
for (int i = 0; i < *count; i++) {
array[i] = atoi(str);
if (str[0] == '-') { // 如果是负数处理特殊字符 '-'
array[i] = -array[i];
}
str = end + 1;
}
return array;
}
// 删除值为x的元素
void removeX(int* arr, int size, int x) {
int j = 0; // 新数组的索引
for (int i = 0; i < size; i++) {
if (arr[i] != x) {
arr[j++] = arr[i]; // 移动非x元素
}
}
arr[j] = 0; // 设置新数组结尾为0,表示已删除所有x
free(arr); // 释放原数组内存
arr = (int*)realloc(arr, j * sizeof(int)); // 重新分配大小
size = j;
}
// 示例主程序
int main() {
char input[] = "1,2,3,x,4,x,5";
int count;
int* arr = stringToIntArray(input, &count);
removeX(arr, count, 3); // 指定要删除的值为3
printf("After removing value %d: ", 3);
for (int i = 0; i < count; i++) {
printf("%d, ", arr[i]);
}
return 0;
}
```
阅读全文
相关推荐
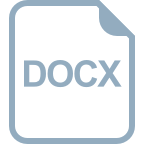
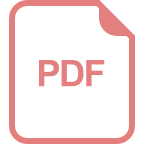
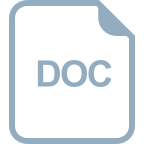

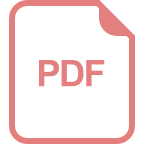
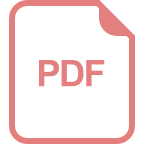
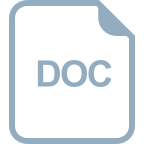
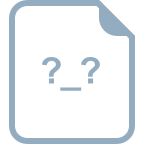
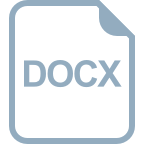
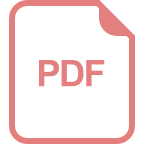
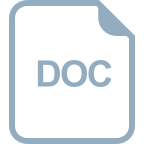
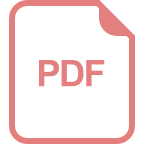
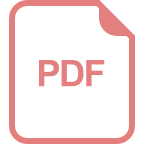
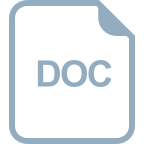
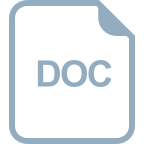
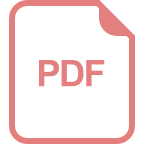
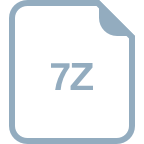