pycharm跑alexnet
时间: 2023-12-22 20:29:39 浏览: 96
在PyCharm中运行AlexNet可以按照以下步骤进行操作:
1. 首先,确保你已经安装了PyTorch和torchvision库。你可以使用以下命令在终端中安装它们:
```shell
pip install torch torchvision
```
2. 在PyCharm中创建一个新的Python项目。
3. 导入必要的库:
```python
import torch
import torchvision.models as models
import torchvision.transforms as transforms
from PIL import Image
```
4. 加载AlexNet模型:
```python
model = models.alexnet(pretrained=True)
```
5. 加载并预处理图像:
```python
image_path = "path_to_your_image.jpg" # 替换为你的图像路径
image = Image.open(image_path)
preprocess = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
input_tensor = preprocess(image)
input_batch = input_tensor.unsqueeze(0)
```
6. 将图像输入模型并获取预测结果:
```python
model.eval()
with torch.no_grad():
output = model(input_batch)
```
7. 解析预测结果:
```python
_, predicted_idx = torch.max(output, 1)
```
8. 打印预测结果:
```python
print("Predicted class index:", predicted_idx.item())
```
请注意,上述代码中的"path_to_your_image.jpg"应替换为你要测试的图像的实际路径。
阅读全文
相关推荐
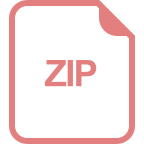
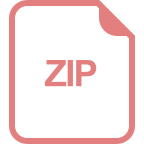
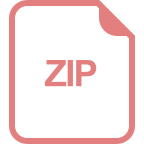
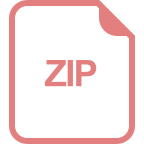
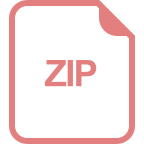
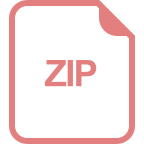
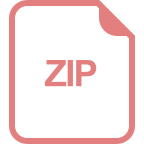
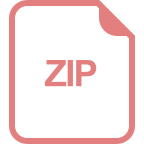
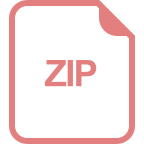
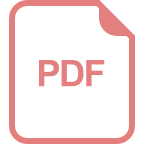
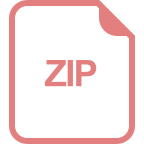
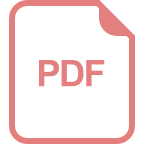
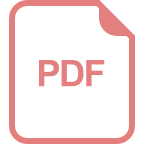