如何使用Arduino设计并实现一个矩阵键盘控制的四则运算计算器,同时将计算结果显示在LCD液晶屏上?请提供详细的编程代码示例以及必要的硬件连接步骤。
时间: 2024-11-29 22:39:04 浏览: 54
设计一个基于Arduino的矩阵键盘控制四则运算计算器,我们需要以下几个步骤:
**硬件连接:**
1. **Arduino Uno**: 连接你的Arduino板到电脑上。
2. **LCD液晶屏**: 按照16x2或16x4这样的尺寸,通过I2C接口连接到数字I/O引脚(如A0-A5),VSS(地)、VDD(电源)。
3. **矩阵键盘**: 将每个按键连接到Arduino的数字引脚,行线接到Arduino的数字I/O口,列线接到另一个I/O口。例如,你可以使用Pins 2-7作为行,Pins 8-13作为列。
**软件编程:**
1. 首先安装必要的库:
- 对于LCD,你需要安装LiquidCrystal库:`#include <LiquidCrystal.h>`
- 对于矩阵键盘,可以使用如MatrixKeypad库:`#include <Keypad.h>`
2. 定义LCD和矩阵键盘实例:
```cpp
LiquidCrystal lcd(12, 11, 5, 4, 3, 2); // LCD I2C pins (if using a 16x2)
Keypad keypad = Keypad(makeKeymap(matrix), makeKeys(row_pins, col_pins)); // Replace 'matrix' and 'row_pins'/'col_pins' with your key matrix and pin arrays
```
3. 编写函数处理按键输入,比如检测用户按下哪个数或运算符:
```cpp
int getKeypress() {
int row = keypad.getKey();
if (row != NO_KEY) {
return keypad.keyMap[row][keypad.keysyms[row].number];
}
return 0; // No key pressed
}
```
4. 主程序循环中处理按键输入,读取用户的运算符、数字,然后进行计算:
```cpp
while (true) {
char input = getKeypress();
switch (input) {
case '1': lcd.setCursor(0, 0); lcd.print("1"); break;
// ... similar cases for other digits and operators like '+', '-', '*', '/'
default:
break; // Ignore any non-digit or operator key
}
// Perform calculation when all four numbers are entered
if (/* Check if all numbers are on the screen */) {
int num1 = /* Get number from LCD */;
int num2 = /* Get another number */;
int result;
switch (input) {
case '+':
result = num1 + num2;
break;
// ... handle other operations
}
lcd.clear();
lcd.print("Result: ");
lcd.print(result);
}
}
```
阅读全文
相关推荐








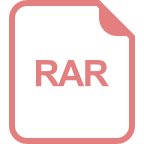





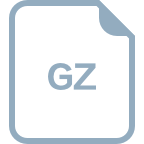


