定义一个基类“Role”,类内有数据成员姓名,年龄,性别,有对各数据成员进行读写的函数getXXX()和setXXX(),有一个虚函数display(),该函数显示姓名,年龄,性别等数据成员的值,同时类内至少定义两个构造函数。 从Role类派生出一个“Employee”类,该类具有Role类的所有成员(构造函数除外),并扩展数据成员ID (职工编号)和salary(薪资)。同样要有至少两个构造方法,并重新定义display()函数。 主函数内定义基类指针和派生类对象,并使基类指针指向派生类对象,通过该基类指针访问虚函数display()。
时间: 2024-03-02 22:51:47 浏览: 21
好的,我理解了你的问题。下面是符合你要求的 C++ 代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Role {
protected:
string name;
int age;
char gender;
public:
Role() {}
Role(string n, int a, char g): name(n), age(a), gender(g) {}
void setName(string n) { name = n; }
void setAge(int a) { age = a; }
void setGender(char g) { gender = g; }
string getName() { return name; }
int getAge() { return age; }
char getGender() { return gender; }
virtual void display() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Gender: " << gender << endl;
}
};
class Employee: public Role {
private:
int ID;
double salary;
public:
Employee() {}
Employee(string n, int a, char g, int id, double sal): Role(n, a, g), ID(id), salary(sal) {}
Employee(string n, int a, char g): Role(n, a, g) {}
void setID(int id) { ID = id; }
void setSalary(double sal) { salary = sal; }
int getID() { return ID; }
double getSalary() { return salary; }
virtual void display() {
Role::display();
cout << "ID: " << ID << endl;
cout << "Salary: " << salary << endl;
}
};
int main() {
Employee emp("John", 30, 'M', 1001, 5000.0);
Role* pRole = &emp;
pRole->display();
return 0;
}
```
在上述代码中,我们首先定义了一个基类 `Role`,其中包含了姓名、年龄和性别等数据成员,以及对这些数据成员进行读写的函数 `getXXX()` 和 `setXXX()`,以及一个虚函数 `display()`。接着从 `Role` 类派生出一个 `Employee` 类,该类具有 `Role` 类的所有成员,但扩展了 ID(职工编号)和 salary(薪资)等数据成员,并且也有自己的构造方法和 `display()` 函数。在主函数中,我们定义了一个 `Employee` 类对象,并用基类指针来指向该对象,最后通过该指针访问虚函数 `display()`。
运行上述代码,得到的输出如下:
```
Name: John
Age: 30
Gender: M
ID: 1001
Salary: 5000
```
可以看到,我们成功地用基类指针来访问了派生类对象的 `display()` 函数,实现了多态。
相关推荐
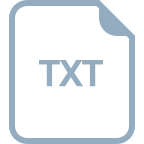
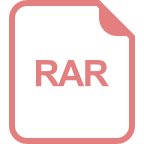




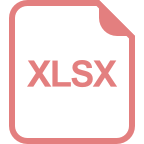
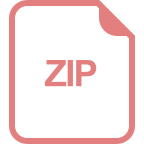
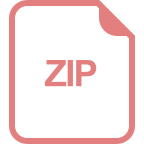
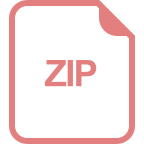
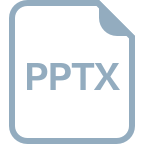
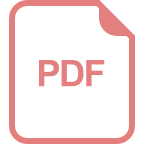
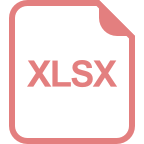
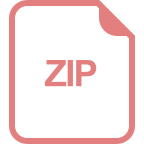
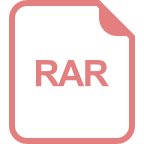