c语言实现贪吃蛇游戏代码
时间: 2023-04-07 10:05:16 浏览: 144
我可以回答这个问题。以下是一个简单的C语言实现贪吃蛇游戏的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 20
#define HEIGHT 20
int snake[100][2];
int food[2];
int length = 3;
int score = 0;
int direction = 3; // 1-up, 2-down, 3-left, 4-right
void gotoxy(int x, int y) {
COORD pos = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void init() {
snake[0][0] = 5;
snake[0][1] = 5;
snake[1][0] = 5;
snake[1][1] = 6;
snake[2][0] = 5;
snake[2][1] = 7;
food[0] = rand() % WIDTH;
food[1] = rand() % HEIGHT;
}
void draw() {
system("cls");
for (int i = 0; i < WIDTH + 2; i++) {
printf("#");
}
printf("\n");
for (int i = 0; i < HEIGHT; i++) {
printf("#");
for (int j = 0; j < WIDTH; j++) {
if (i == snake[0][0] && j == snake[0][1]) {
printf("O");
} else if (i == food[0] && j == food[1]) {
printf("F");
} else {
int is_body = 0;
for (int k = 1; k < length; k++) {
if (i == snake[k][0] && j == snake[k][1]) {
printf("o");
is_body = 1;
break;
}
}
if (!is_body) {
printf(" ");
}
}
}
printf("#\n");
}
for (int i = 0; i < WIDTH + 2; i++) {
printf("#");
}
printf("\n");
printf("Score: %d\n", score);
}
void update() {
int tail[2];
tail[0] = snake[length - 1][0];
tail[1] = snake[length - 1][1];
for (int i = length - 1; i > 0; i--) {
snake[i][0] = snake[i - 1][0];
snake[i][1] = snake[i - 1][1];
}
if (direction == 1) {
snake[0][0]--;
} else if (direction == 2) {
snake[0][0]++;
} else if (direction == 3) {
snake[0][1]--;
} else if (direction == 4) {
snake[0][1]++;
}
if (snake[0][0] == food[0] && snake[0][1] == food[1]) {
length++;
score += 10;
food[0] = rand() % WIDTH;
food[1] = rand() % HEIGHT;
}
if (snake[0][0] < 0 || snake[0][0] >= HEIGHT || snake[0][1] < 0 || snake[0][1] >= WIDTH) {
printf("Game over!\n");
exit(0);
}
for (int i = 1; i < length; i++) {
if (snake[0][0] == snake[i][0] && snake[0][1] == snake[i][1]) {
printf("Game over!\n");
exit(0);
}
}
}
int main() {
init();
while (1) {
draw();
update();
Sleep(100);
if (_kbhit()) {
int c = _getch();
if (c == 'w' && direction != 2) {
direction = 1;
} else if (c == 's' && direction != 1) {
direction = 2;
} else if (c == 'a' && direction != 4) {
direction = 3;
} else if (c == 'd' && direction != 3) {
direction = 4;
}
}
}
return 0;
}
```
相关推荐
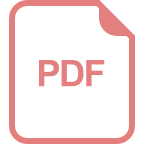
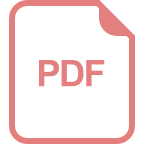
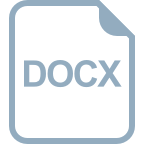
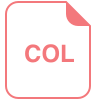
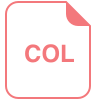
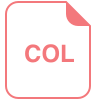
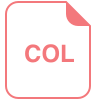
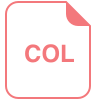









