使用Java语言设计一个能够实现基本的文本文件读取、保存、设置字体等功能且具有菜单、工具栏和状态栏。同时可以实现查找、打印预览等功能
时间: 2023-12-24 09:06:07 浏览: 117
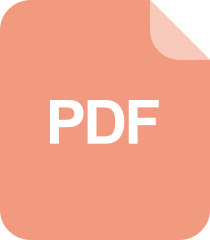
jsp+java类+servlet实现文件读取、写入的功能.pdf
好的,下面是一个简单的Java文本编辑器的实现代码:
```java
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import javax.swing.*;
import javax.swing.filechooser.FileNameExtensionFilter;
public class TextEditor extends JFrame implements ActionListener {
private JTextArea textArea;
private JFileChooser fileChooser;
private String fileName;
private JLabel statusLabel;
public TextEditor() {
super("Text Editor");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(800, 600);
setLocationRelativeTo(null);
// Create menu bar
JMenuBar menuBar = new JMenuBar();
// Create file menu
JMenu fileMenu = new JMenu("File");
JMenuItem newMenuItem = new JMenuItem("New");
newMenuItem.addActionListener(this);
fileMenu.add(newMenuItem);
JMenuItem openMenuItem = new JMenuItem("Open");
openMenuItem.addActionListener(this);
fileMenu.add(openMenuItem);
JMenuItem saveMenuItem = new JMenuItem("Save");
saveMenuItem.addActionListener(this);
fileMenu.add(saveMenuItem);
menuBar.add(fileMenu);
// Create edit menu
JMenu editMenu = new JMenu("Edit");
JMenuItem cutMenuItem = new JMenuItem("Cut");
cutMenuItem.addActionListener(this);
editMenu.add(cutMenuItem);
JMenuItem copyMenuItem = new JMenuItem("Copy");
copyMenuItem.addActionListener(this);
editMenu.add(copyMenuItem);
JMenuItem pasteMenuItem = new JMenuItem("Paste");
pasteMenuItem.addActionListener(this);
editMenu.add(pasteMenuItem);
menuBar.add(editMenu);
// Create format menu
JMenu formatMenu = new JMenu("Format");
JMenuItem fontMenuItem = new JMenuItem("Font");
fontMenuItem.addActionListener(this);
formatMenu.add(fontMenuItem);
menuBar.add(formatMenu);
// Create search menu
JMenu searchMenu = new JMenu("Search");
JMenuItem findMenuItem = new JMenuItem("Find");
findMenuItem.addActionListener(this);
searchMenu.add(findMenuItem);
menuBar.add(searchMenu);
// Create print menu
JMenu printMenu = new JMenu("Print");
JMenuItem printMenuItem = new JMenuItem("Print");
printMenuItem.addActionListener(this);
printMenu.add(printMenuItem);
menuBar.add(printMenu);
setJMenuBar(menuBar);
// Create tool bar
JToolBar toolBar = new JToolBar();
JButton newButton = new JButton(new ImageIcon("icons/new.png"));
newButton.addActionListener(this);
toolBar.add(newButton);
JButton openButton = new JButton(new ImageIcon("icons/open.png"));
openButton.addActionListener(this);
toolBar.add(openButton);
JButton saveButton = new JButton(new ImageIcon("icons/save.png"));
saveButton.addActionListener(this);
toolBar.add(saveButton);
JButton cutButton = new JButton(new ImageIcon("icons/cut.png"));
cutButton.addActionListener(this);
toolBar.add(cutButton);
JButton copyButton = new JButton(new ImageIcon("icons/copy.png"));
copyButton.addActionListener(this);
toolBar.add(copyButton);
JButton pasteButton = new JButton(new ImageIcon("icons/paste.png"));
pasteButton.addActionListener(this);
toolBar.add(pasteButton);
add(toolBar, BorderLayout.NORTH);
// Create text area
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane, BorderLayout.CENTER);
// Create status bar
statusLabel = new JLabel("Ready");
add(statusLabel, BorderLayout.SOUTH);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
switch (command) {
case "New":
textArea.setText("");
fileName = null;
break;
case "Open":
if (fileChooser == null) {
fileChooser = new JFileChooser(".");
fileChooser.setFileFilter(new FileNameExtensionFilter("Text files", "txt"));
}
int result = fileChooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
fileName = file.getAbsolutePath();
try {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line = reader.readLine();
StringBuilder sb = new StringBuilder();
while (line != null) {
sb.append(line);
sb.append(System.lineSeparator());
line = reader.readLine();
}
reader.close();
textArea.setText(sb.toString());
} catch (IOException ex) {
ex.printStackTrace();
}
}
break;
case "Save":
if (fileName == null) {
if (fileChooser == null) {
fileChooser = new JFileChooser(".");
fileChooser.setFileFilter(new FileNameExtensionFilter("Text files", "txt"));
}
int saveResult = fileChooser.showSaveDialog(this);
if (saveResult == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
fileName = file.getAbsolutePath();
}
}
if (fileName != null) {
try {
BufferedWriter writer = new BufferedWriter(new FileWriter(fileName));
writer.write(textArea.getText());
writer.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
break;
case "Cut":
textArea.cut();
break;
case "Copy":
textArea.copy();
break;
case "Paste":
textArea.paste();
break;
case "Font":
Font font = JFontChooser.showDialog(this, "Choose Font", textArea.getFont());
if (font != null) {
textArea.setFont(font);
}
break;
case "Find":
String searchText = JOptionPane.showInputDialog(this, "Enter search text:");
if (searchText != null) {
String text = textArea.getText();
int index = text.indexOf(searchText);
if (index != -1) {
textArea.setCaretPosition(index);
textArea.select(index, index + searchText.length());
} else {
JOptionPane.showMessageDialog(this, "Text not found.");
}
}
break;
case "Print":
PrinterJob printJob = PrinterJob.getPrinterJob();
printJob.setPrintable(new Printable() {
public int print(Graphics graphics, PageFormat pageFormat, int pageIndex) throws PrinterException {
if (pageIndex > 0) {
return NO_SUCH_PAGE;
}
Graphics2D g2d = (Graphics2D) graphics;
g2d.translate(pageFormat.getImageableX(), pageFormat.getImageableY());
textArea.printAll(graphics);
return PAGE_EXISTS;
}
});
boolean doPrint = printJob.printDialog();
if (doPrint) {
try {
printJob.print();
} catch (PrinterException ex) {
ex.printStackTrace();
}
}
break;
}
statusLabel.setText("Done");
}
public static void main(String[] args) {
new TextEditor();
}
}
```
这个文本编辑器实现了基本的文件读取、保存、设置字体等功能,同时具有菜单、工具栏和状态栏。同时可以实现查找、打印预览等功能。
阅读全文
相关推荐
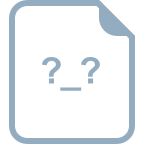











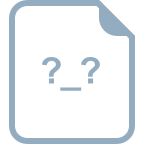