ubuntu c语言 多线程编程 案例
时间: 2024-07-06 20:00:43 浏览: 219
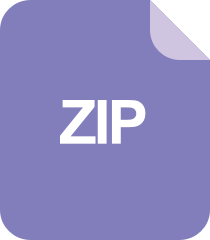
C语言彩票管理系统源码
在Ubuntu系统上使用C语言进行多线程编程是一个常见的实践,因为C库提供了`pthread.h`头文件,支持POSIX标准线程API。以下是一个简单的多线程C语言案例:
```c
#include <stdio.h>
#include <pthread.h>
// 定义共享数据
int shared_data = 0;
// 共享数据更新函数
void* increment_data(void* arg) {
for (int i = 0; i < 1000; i++) {
shared_data++;
}
printf("Thread %d updated shared data to: %d\n", pthread_self(), shared_data);
return NULL;
}
int main() {
// 创建两个线程
pthread_t thread1, thread2;
int result;
result = pthread_create(&thread1, NULL, increment_data, NULL); // 线程1
if (result != 0) {
perror("Error creating thread 1");
exit(1);
}
result = pthread_create(&thread2, NULL, increment_data, NULL); // 线程2
if (result != 0) {
perror("Error creating thread 2");
exit(1);
}
// 等待线程执行完毕
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
printf("Main thread: Shared data after threads finish: %d\n", shared_data);
return 0;
}
```
在这个例子中,我们创建了两个线程(`increment_data`),它们分别更新共享的`shared_data`变量。`pthread_create`用于创建新线程,`pthread_join`用于等待线程执行完成。注意,在这个例子中没有使用互斥锁(mutex)来保护对共享数据的访问,实际应用中需要考虑同步机制以避免数据竞争。
阅读全文
相关推荐
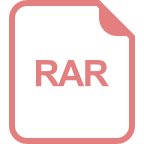
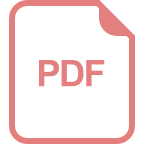
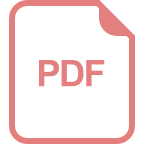
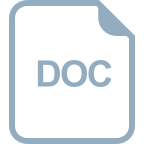
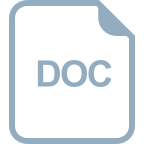
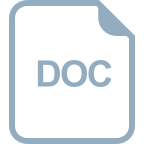
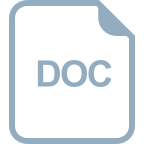
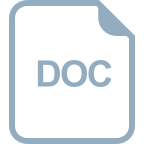
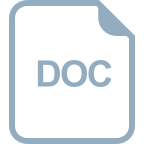
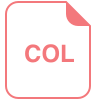
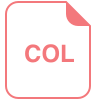
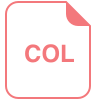
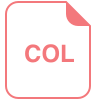
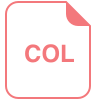
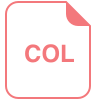
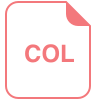
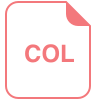
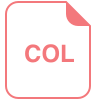