用python爬虫在京东抢购茅台(严谨版)源码
时间: 2023-09-01 10:04:41 浏览: 141
使用Python爬虫在京东抢购茅台的源码如下:
```python
import time
import random
import requests
from selenium import webdriver
def jd_login(username, password):
driver = webdriver.Chrome() # 需要安装并配置Chrome浏览器驱动
driver.get('https://passport.jd.com/new/login.aspx')
driver.find_element_by_link_text('账户登录').click()
driver.find_element_by_id('loginname').send_keys(username)
driver.find_element_by_id('nloginpwd').send_keys(password)
driver.find_element_by_id('loginsubmit').click()
time.sleep(5)
if driver.current_url != 'https://www.jd.com/':
print("登录失败,请检查账号密码是否正确")
driver.quit()
return None
print("登录成功")
return driver
def jd_buy(driver, url):
driver.get(url)
time.sleep(random.uniform(0.1, 0.5))
driver.find_element_by_id('InitCartUrl').click() # 将商品加入购物车
time.sleep(random.uniform(0.1, 0.5))
driver.get('https://cart.jd.com/cart') # 跳转到购物车页面
time.sleep(random.uniform(0.1, 0.5))
if driver.current_url != 'https://cart.jd.com/cart':
print("购买失败,请检查商品链接是否正确")
driver.quit()
return
driver.find_element_by_id('toggle-checkboxes_up').click() # 全选购物车中的商品
time.sleep(1)
driver.find_element_by_link_text('去结算').click() # 结算
time.sleep(1)
driver.find_element_by_link_text('提交订单').click() # 提交订单
print("抢购成功!请尽快完成支付")
if __name__ == '__main__':
username = 'Your-Username' # 替换为京东账号的用户名
password = 'Your-Password' # 替换为京东账号的密码
login_url = 'https://passport.jd.com/new/login.aspx'
product_url = 'https://item.jd.com/100008348085.html' # 替换为茅台商品的链接
driver = jd_login(username, password)
if driver:
jd_buy(driver, product_url)
```
注意事项:
1. 需要先安装并配置好Chrome浏览器驱动。
2. 需要将代码中的`Your-Username`和`Your-Password`替换为正确的京东账号用户名和密码。
3. 需要将代码中的`https://item.jd.com/100008348085.html`替换为茅台商品的正确链接。
4. 该代码仅供学习和参考,使用爬虫进行抢购有一定风险,并非鼓励违反京东规则,使用需谨慎。
相关推荐
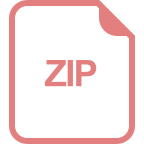
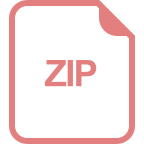
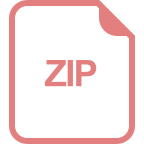
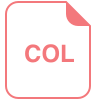
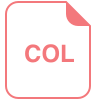
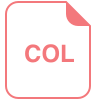
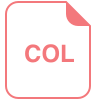
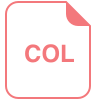









