给定两个字符串 s 和 t ,编写一个函数来判断 t 是否是 s 的字母异位词。 注意:若 s 和 t 中每个字符出现的次数都相同,则称 s 和 t 互为字母异位词。 用c语言解决上述问题
时间: 2024-10-05 14:04:40 浏览: 34
在C语言中,我们可以创建一个函数`isAnagram(s[], t[])`来判断两个字符串`s`和`t`是否为字母异位词。这个函数的基本思路是先检查两个字符串的长度是否相等,因为如果长度不同,它们就不可能是异位词。然后,我们对两个字符串的字符进行计数,并比较计数结果。
以下是一个简单的实现示例:
```c
#include <stdio.h>
#include <string.h>
int isAnagram(char s[], char t[]) {
int len_s = strlen(s);
int len_t = strlen(t);
// 如果长度不相等,直接返回0
if (len_s != len_t) {
return 0;
}
// 定义一个大小为26的数组来存储字符计数
int count[26] = {0};
// 遍历s并增加对应字符的计数
for (int i = 0; i < len_s; ++i) {
count[s[i] - 'a']++;
}
// 再遍历t,减去对应字符的计数,如果发现有负数说明不是异位词
for (int i = 0; i < len_t; ++i) {
count[t[i] - 'a']--;
if (count[t[i] - 'a'] < 0) {
return 0;
}
}
// 检查所有字符计数是否都为0,如果是则返回1表示是异位词
for (int i = 0; i < 26; ++i) {
if (count[i] != 0) {
return 0;
}
}
return 1;
}
int main() {
char s[] = "anagram";
char t[] = "nagaram";
if (isAnagram(s, t)) {
printf("%s and %s are anagrams.\n", s, t);
} else {
printf("%s and %s are not anagrams.\n", s, t);
}
return 0;
}
```
阅读全文
相关推荐
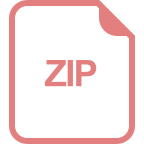
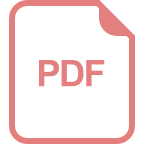



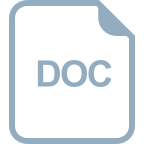
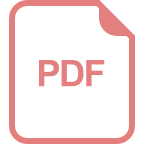
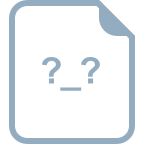
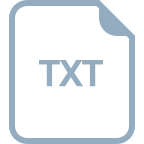
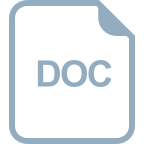
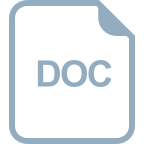
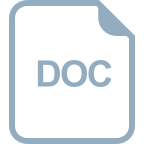
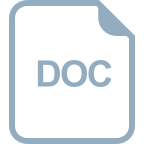
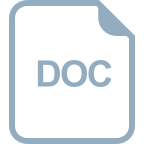
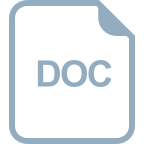
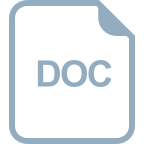
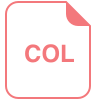
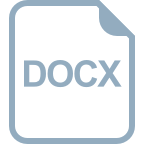