python编写选择题程序显示图片
时间: 2023-08-08 20:08:23 浏览: 182
以下是一个简单的 Python 代码示例,可以实现显示图片和选择题功能:
```python
from PIL import Image
from io import BytesIO
# 定义题目和选项
question = "这是哪个国家的国旗?"
options = ["中国", "美国", "英国", "法国"]
# 加载图片
image_url = "https://example.com/flag.jpg"
image_response = requests.get(image_url)
image = Image.open(BytesIO(image_response.content))
image.show()
# 显示题目和选项
print(question)
for i in range(len(options)):
print(f"{i+1}. {options[i]}")
# 获取用户选择
answer = int(input("请输入答案选项的编号:"))
# 判断答案是否正确
if answer == 1:
print("回答正确!")
else:
print("回答错误!")
```
这个程序使用了 Python 的 Pillow 库来加载和显示图片,使用 requests 库来获取图片的二进制数据。用户可以通过输入选项的编号来进行选择,程序会判断用户的答案是否正确并给出相应的提示。
相关问题
python编写选择题程序显示已经有的图片
以下是一个简单的 Python 代码示例,可以实现显示已经有的图片和选择题功能:
```python
from PIL import Image
# 定义题目和选项
question = "这是哪个国家的国旗?"
options = ["中国", "美国", "英国", "法国"]
# 加载图片
image_path = "path/to/flag.jpg"
image = Image.open(image_path)
image.show()
# 显示题目和选项
print(question)
for i in range(len(options)):
print(f"{i+1}. {options[i]}")
# 获取用户选择
answer = int(input("请输入答案选项的编号:"))
# 判断答案是否正确
if answer == 1:
print("回答正确!")
else:
print("回答错误!")
```
这个程序使用了 Python 的 Pillow 库来加载和显示图片。用户可以通过输入选项的编号来进行选择,程序会判断用户的答案是否正确并给出相应的提示。在代码中,你需要将 `path/to/flag.jpg` 替换成你具体的图片路径。
python编写选择题程序
以下是一个简单的Python选择题程序的示例代码:
```python
questions = [
{
"question": "What is the capital of France?",
"options": ["London", "Paris", "Berlin", "Madrid"],
"answer": "Paris"
},
{
"question": "What is the largest planet in our solar system?",
"options": ["Jupiter", "Saturn", "Neptune", "Mercury"],
"answer": "Jupiter"
},
{
"question": "What is the smallest country in the world?",
"options": ["Monaco", "Vatican City", "San Marino", "Liechtenstein"],
"answer": "Vatican City"
}
]
score = 0
for q in questions:
print(q["question"])
for i, option in enumerate(q["options"]):
print(f"{i+1}. {option}")
user_answer = input("Your answer: ")
if user_answer.lower() == q["answer"].lower():
print("Correct!")
score += 1
else:
print("Incorrect!")
print(f"Final score: {score}/{len(questions)}")
```
在上面的代码中,我们首先定义了一个包含三个问题的列表,每个问题都包含问题、选项和答案。然后,我们使用一个for循环遍历每个问题,并打印问题和选项。用户可以输入他们的答案,然后我们检查它是否与正确的答案匹配。最后,我们打印出用户的最终得分。
请注意,上面的代码只是一个简单的示例,可以根据需要进行修改或扩展。
阅读全文
相关推荐
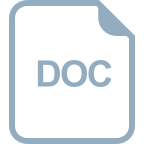



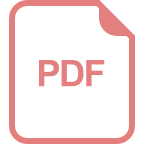
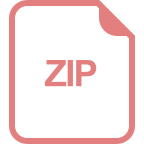
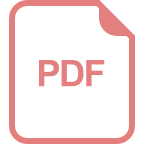
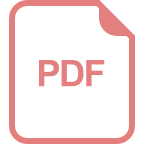
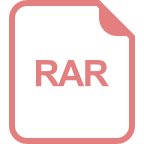
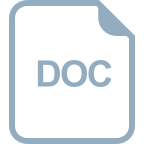
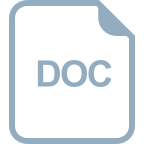
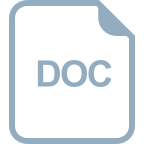
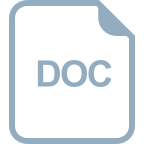

