如何将tuple里的张量分别传递给cuda设备
时间: 2024-04-10 16:28:32 浏览: 48
要将元组(tuple)中的张量分别传递给CUDA设备,你可以使用`torch.device()`和`.to()`方法。以下是一个示例:
```python
import torch
# 创建一个包含张量的元组
tensors = (torch.tensor([1, 2, 3]), torch.tensor([4, 5, 6]))
# 将元组中的张量分别传递给CUDA设备
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
tensors = tuple(tensor.to(device) for tensor in tensors)
# 打印每个张量所在的设备
for tensor in tensors:
print(tensor.device)
```
在上面的示例中,我们首先创建一个包含两个张量的元组。然后,我们使用生成器表达式遍历元组中的每个张量,并将它们分别传递给CUDA设备。最后,我们遍历更新后的元组,并打印每个张量所在的设备。
这样,你就可以将元组中的张量分别传递给CUDA设备了。请注意,生成器表达式用于在迭代过程中创建更新后的元组。
相关问题
怎样将list中的tuple传入cuda
要将list中的tuple传入cuda,需要先将其转换为torch tensor。可以使用以下代码将list中的tuple转换为tensor并放入cuda中:
```python
import torch
# 创建list of tuple
my_list = [(1, 2), (3, 4), (5, 6)]
# 将list of tuple转换为tensor
my_tensor = torch.tensor(my_list)
# 将tensor放入cuda
my_tensor = my_tensor.cuda()
```
在这个例子中,`my_list` 是一个包含三个元素的list,每个元素都是一个二元组。将`my_list`转换为tensor时,每个二元组会被转换为一个长度为2的一维tensor。最终得到的`my_tensor`是一个3x2的二维tensor。
如果tuple中含有多个元素,则需要使用`torch.tensor()`中的`dim`参数指定每个维度的大小。例如:
```python
import torch
# 创建list of tuple
my_list = [((1, 2), (3, 4)), ((5, 6), (7, 8)), ((9, 10), (11, 12))]
# 将list of tuple转换为tensor
my_tensor = torch.tensor(my_list, dim=2)
# 将tensor放入cuda
my_tensor = my_tensor.cuda()
```
在这个例子中,`my_list` 是一个包含三个元素的list,每个元素都是一个二元组,每个二元组都包含两个二元组。将`my_list`转换为tensor时,每个元组会被转换为一个长度为2的一维tensor,每个二元组会被转换为一个长度为2的一维tensor。最终得到的`my_tensor`是一个3x2x2的三维tensor。
'tuple' object has no attribute 'cuda'
### 回答1:
这个错误是因为你在尝试将一个元组对象(tuple)传递给CUDA设备时,但元组对象没有cuda属性。可能是你想将一个包含多个张量的元组传递给CUDA设备,但是你需要将每个张量都分别传递给CUDA设备,而不是整个元组。
### 回答2:
这个错误信息通常发生在使用PyTorch训练模型时出现。它的意思是说在一个元组(tuple)对象上尝试使用CUDA(即使用GPU来加速运算),但是元组并没有相应的CUDA属性。
通常这种错误发生在网络的正向传播过程中,可能是由于模型的某些部分没有被正确地移动到GPU上,或者是由于没有正确地将输入数据移动到GPU上。通常情况下,模型的参数需要被明确地转移到GPU上进行计算。
解决这个问题有几种方法。第一种方法是检查是否在定义模型时使用了`.cuda()`或`.to()`来将模型参数显式地移动到GPU上。如果没有,可以尝试在训练过程中添加这些语句。
第二种方法是确保输入数据也被正确地移动到GPU上。可以使用`.cuda()`或`.to()`来将输入数据移动到GPU上。
第三种方法是检查代码中是否有错误,例如在某个位置错误地将元组赋值给了模型参数,或者使用了错误的对象类型。
在任何一种方法中,最重要的是要仔细检查代码并确认所有对象被正确地移动到GPU上。这样才能避免发生“'tuple' object has no attribute 'cuda'”这种错误。
### 回答3:
“tuple" object has no attribute "cuda"这个错误通常是由于在将Tuple中的数据传递给PyTorch的GPU时出现了问题导致的。在PyTorch中,tuple(元组)对象不能被转换为GPU数据类型,因为tuple是不可变的对象,不能在GPU上运行。只有可变的对象,如Tensor,Variable等,才能在GPU上运行。
如果您正在使用PyTorch进行深度学习模型的编写,那么在使用元组时,要格外小心。元组通常用于将多个变量捆绑在一起,这样可以传递多个变量。但是,在使用PyTorch时,应该考虑到它对GPU的支持,因此,将元组传递给GPU时,需要先将元组中的Tensor转换为Variable,然后再将Variable传递给GPU。
如果在代码中出现了"tuple" object has no attribute "cuda"的错误,则需要检查代码中是否使用了元组,并检查元组中是否有Tensor对象。如果是Tensor对象,则需要将它们转换为Variable对象,并将Variable对象传递给GPU。如果不是,那么错误的原因可能是其他问题,需要仔细检查代码中的错误。
总之,"tuple" object has no attribute "cuda"的错误是PyTorch代码中常见的错误之一,通常是由于转换Tensor对象时出现问题导致的。检查代码并将Tensor对象转换为Variable对象,然后再将Variable对象传递给GPU,可以帮助解决这个问题。
相关推荐
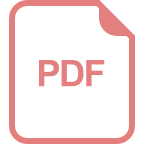
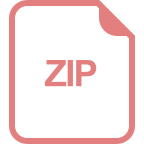
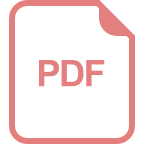












