queue python
时间: 2023-10-21 14:30:33 浏览: 152
A queue is a collection of elements that supports adding elements to the back (enqueue) and removing elements from the front (dequeue). The elements are added to the back of the queue and removed from the front in a first-in-first-out (FIFO) order. In Python, a queue can be implemented using the built-in deque class from the collections module or using the Queue class from the queue module. Queues are commonly used in data structures and algorithms, such as breadth-first search and job scheduling.
相关问题
queue python
在Python中,队列(queue)是一种数据结构,遵循先进先出(FIFO)的原则。Python提供了内置模块`queue`,用于实现队列的操作。
要使用队列,首先需要导入`queue`模块:
```python
import queue
```
然后,可以创建一个队列对象:
```python
q = queue.Queue()
```
可以使用`put()`方法将元素添加到队列中:
```python
q.put(item)
```
使用`get()`方法从队列中获取元素,并将其从队列中删除:
```python
item = q.get()
```
还可以使用`empty()`方法检查队列是否为空:
```python
if q.empty():
print("队列为空")
```
这只是队列操作的基本示例,还有其他方法可用于队列的控制和查询。需要根据具体需求选择合适的队列类型,例如`queue.LifoQueue`(后进先出)或`queue.PriorityQueue`(按优先级排序)。
数据结构queue python
队列(Queue)是一种基本的数据结构,遵循先进先出(FIFO)的原则。在Python中,可以使用列表(List)或者collections模块中的deque(双端队列)来实现队列。
使用列表实现队列时,可以使用append()方法在列表末尾添加元素,并使用pop(0)方法从列表开头移除元素。示例代码如下:
```python
queue = []
queue.append(1) # 入队列
queue.append(2)
queue.append(3)
print(queue) # 输出: [1, 2, 3]
item = queue.pop(0) # 出队列
print(item) # 输出: 1
print(queue) # 输出: [2, 3]
```
使用deque来实现队列时,可以使用append()方法在队列末尾添加元素,并使用popleft()方法从队列开头移除元素,deque是一个双端队列,所以性能更好。示例代码如下:
```python
from collections import deque
queue = deque()
queue.append(1) # 入队列
queue.append(2)
queue.append(3)
print(queue) # 输出: deque([1, 2, 3])
item = queue.popleft() # 出队列
print(item) # 输出: 1
print(queue) # 输出: deque([2, 3])
```
阅读全文
相关推荐
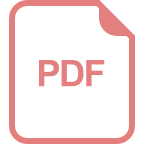
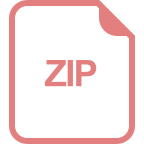









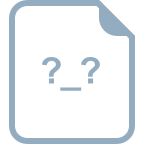
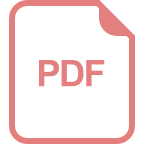
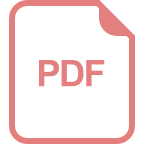
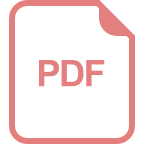
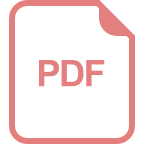