重新设计C语言版本的BST树模块
时间: 2023-06-16 12:05:22 浏览: 69
好的,下面是我重新设计的C语言版本的BST树模块:
```
// bst.h
// 定义二叉搜索树节点类型
typedef struct bst_node {
int key;
struct bst_node* left;
struct bst_node* right;
} BSTNode;
// 创建一个新的节点
BSTNode* create_node(int key);
// 插入一个节点,返回根节点
BSTNode* insert_node(BSTNode* root, int key);
// 删除一个节点,返回根节点
BSTNode* delete_node(BSTNode* root, int key);
// 查找一个节点,返回指向该节点的指针
BSTNode** search_node(BSTNode** root, int key);
// 遍历二叉搜索树,并将节点的key值存储到数组中
void traverse_bst(BSTNode* root, int* arr, int* index);
// 释放二叉搜索树的内存
void free_bst(BSTNode* root);
```
```c
// bst.c
#include "bst.h"
#include <stdlib.h>
// 创建一个新的节点
BSTNode* create_node(int key) {
BSTNode* node = (BSTNode*)malloc(sizeof(BSTNode));
node->key = key;
node->left = NULL;
node->right = NULL;
return node;
}
// 插入一个节点,返回根节点
BSTNode* insert_node(BSTNode* root, int key) {
if (root == NULL) {
return create_node(key);
}
if (key < root->key) {
root->left = insert_node(root->left, key);
} else if (key > root->key) {
root->right = insert_node(root->right, key);
}
return root;
}
// 删除一个节点,返回根节点
BSTNode* delete_node(BSTNode* root, int key) {
if (root == NULL) {
return NULL;
}
if (key < root->key) {
root->left = delete_node(root->left, key);
} else if (key > root->key) {
root->right = delete_node(root->right, key);
} else {
if (root->left == NULL) {
BSTNode* temp = root->right;
free(root);
return temp;
} else if (root->right == NULL) {
BSTNode* temp = root->left;
free(root);
return temp;
}
BSTNode* temp = root->right;
while (temp->left != NULL) {
temp = temp->left;
}
root->key = temp->key;
root->right = delete_node(root->right, temp->key);
}
return root;
}
// 查找一个节点,返回指向该节点的指针
BSTNode** search_node(BSTNode** root, int key) {
if (*root == NULL || (*root)->key == key) {
return root;
}
if (key < (*root)->key) {
return search_node(&((*root)->left), key);
} else {
return search_node(&((*root)->right), key);
}
}
// 遍历二叉搜索树,并将节点的key值存储到数组中
void traverse_bst(BSTNode* root, int* arr, int* index) {
if (root == NULL) {
return;
}
traverse_bst(root->left, arr, index);
arr[*index] = root->key;
(*index)++;
traverse_bst(root->right, arr, index);
}
// 释放二叉搜索树的内存
void free_bst(BSTNode* root) {
if (root == NULL) {
return;
}
free_bst(root->left);
free_bst(root->right);
free(root);
}
```
这个BST树模块包含了创建节点、插入节点、删除节点、查找节点、遍历和释放内存等基本操作。在使用时,可以通过调用这些函数来实现对BST树的操作。
相关推荐
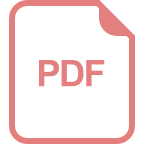
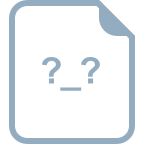
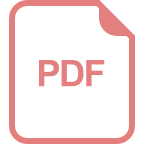














