在C++中不使用OpenCV库的情况下,如何将字母插入到图片中?具体代码实现
时间: 2024-11-29 13:33:31 浏览: 35
在C++中不依赖OpenCV库的话,你可以使用标准库如`std::stringstream`和`std::fstream`来读取图片文件,然后利用位运算或者直接修改像素值的方式将字母插入。这里是一个简单的例子,假设我们已经有了一个图片文件,并且已经处理好字体和颜色:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
// 假设你有一个函数或方法可以生成单个字符的位图
void insert_char(int x, int y, char c, unsigned char* img, int width, int height) {
std::string font_data = "..."; // 字体数据,你需要将其替换为实际的字体数据
for (size_t i = 0; i < font_data.size(); ++i) {
if (c == static_cast<char>(i)) {
// 将字体数据的对应位置复制到图像上
for (int j = 0; j < height; ++j) {
img[(y + j) * width + x] = font_data[i];
}
break;
}
}
}
int main() {
try {
// 打开图片文件并读取二进制数据
std::ifstream image_file("image.jpg", std::ios::binary);
if (!image_file.is_open()) {
std::cerr << "Error opening file." << std::endl;
return -1;
}
// 获取图像尺寸
image_file.seekg(0, std::ios::end);
int size = image_file.tellg();
image_file.seekg(0, std::ios::beg);
unsigned char* img = new unsigned char[size];
image_file.read(img, size);
// 假定图片宽度和高度
int width = ...; // 比如,从头信息获取
int height = ...;
// 插入字符,例如 'A'
insert_char(10, 20, 'A', img, width, height); // 插入坐标和字符
// 写回文件
std::ofstream output_file("output_image.jpg", std::ios::binary);
output_file.write(img, size);
delete[] img;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
请注意,这个示例非常基础,实际应用中可能需要更复杂的图像处理技术来保证插入后的图像质量和兼容性。
阅读全文
相关推荐
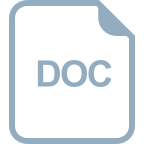
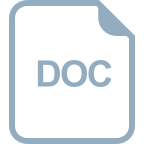
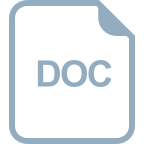
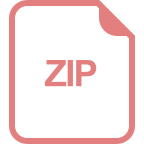
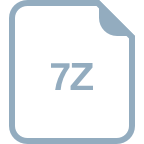
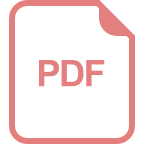
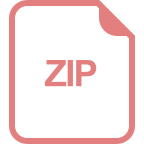
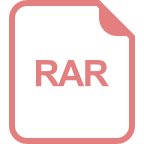
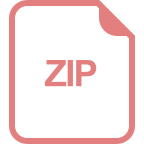
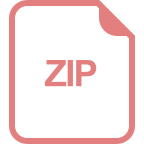
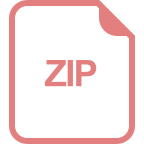
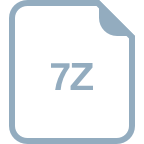
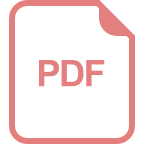
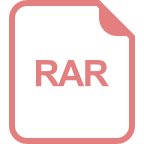
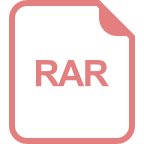
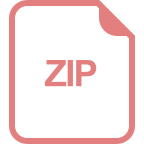