c++ 将set转为list
时间: 2023-10-06 18:05:19 浏览: 107
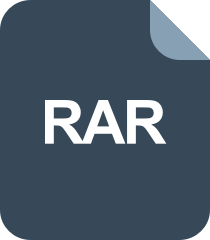
set转成list集合再排序输出

要将一个 `std::set` 转换为 `std::list`,可以使用 `std::copy` 算法和 `std::back_inserter` 迭代器,如下所示:
```cpp
#include <set>
#include <list>
#include <algorithm>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
std::list<int> myList;
std::copy(mySet.begin(), mySet.end(), std::back_inserter(myList));
return 0;
}
```
这里将 `mySet` 的元素复制到 `myList` 中,`std::back_inserter` 是一个迭代器适配器,将插入操作转换为 `push_back` 操作。
阅读全文
相关推荐
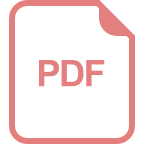
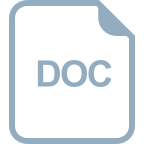
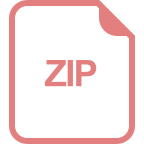
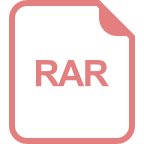
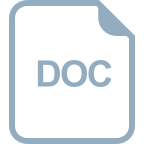
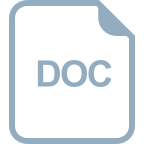
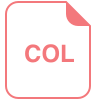

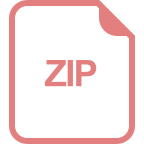
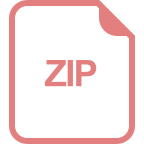
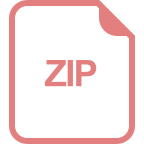
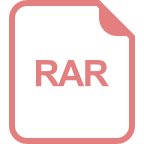
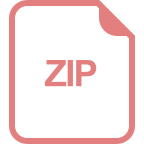
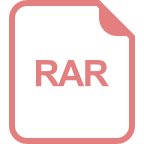