java 使用ApplicationContext.getBean获取Bean对象示例
时间: 2024-02-28 14:55:11 浏览: 142
好的,关于您的问题,可以使用以下方式获取Bean对象实例:
1.通过ApplicationContext的getBean方法获取:
```java
//通过Class对象获取
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Person person = context.getBean(Person.class);
//通过id获取
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Person person = (Person)context.getBean("person");
```
2.通过注解@Autowired获取:
```java
@Service
public class PersonService {
@Autowired
private PersonRepository personRepository;
}
```
这里的@Autowired注解会自动帮助我们注入PersonRepository的实例。需要注意的是,这种方式需要在Spring配置文件中配置扫描注解的包路径,以便Spring能够扫描并注入相应的实例。
希望这个回答能够帮到您。
相关问题
applicationcontext.getbean()的作用是什么啊
`applicationContext.getBean()`方法的作用是从 Spring 容器中获取一个已经注册的 bean 实例。
在 Spring 框架中,我们通常使用依赖注入的方式来管理和使用 bean。`getBean()`方法是用来手动获取 bean 实例的方式,可以在需要的地方直接获取指定的 bean 对象。
该方法接受一个参数,即需要获取的 bean 的名称或类型。如果传入的是名称,那么该方法将返回与该名称对应的 bean 实例;如果传入的是类型,那么该方法将返回与该类型匹配的 bean 实例。如果找不到对应的 bean,会抛出异常。
需要注意的是,在使用`getBean()`方法之前,需要确保 Spring 容器已经初始化完毕,并且已经注册了需要获取的 bean。
示例:
```java
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
MyBean myBean = (MyBean) applicationContext.getBean("myBean");
```
在上面的例子中,通过`applicationContext.getBean("myBean")`获取了名为 "myBean" 的 bean 实例,并将其转换为 `MyBean` 类型。
ApplicationContextUtil.getBean
ApplicationContext.getBean 是一个静态方法,用于获取 Spring 容器中指定名称的 Bean 实例。该方法通常用于在非 Spring 管理的类中获取 Spring 管理的 Bean 实例。
下面是一个示例:
```java
@Service
public class UserService {
public void login() {
// ...
}
}
public class LoginController {
public void handleLogin() {
UserService userService = ApplicationContextUtil.getBean("userService", UserService.class);
userService.login();
}
}
public class ApplicationContextUtil implements ApplicationContextAware {
private static ApplicationContext context;
@Override
public void setApplicationContext(ApplicationContext context) throws BeansException {
ApplicationContextUtil.context = context;
}
public static <T> T getBean(String name, Class<T> clazz) {
return context.getBean(name, clazz);
}
}
```
在上面的代码中,LoginController 类不是 Spring 管理的 Bean,但是它需要使用 UserService 类,因此使用 ApplicationContextUtil.getBean 方法获取 UserService 的实例。注意,ApplicationContextUtil 类需要实现 ApplicationContextAware 接口,并在 setApplicationContext 方法中保存 ApplicationContext 实例,以便后续使用。
阅读全文
相关推荐
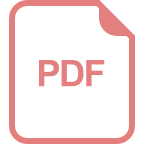
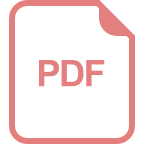
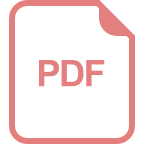
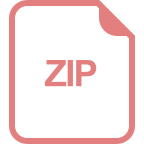
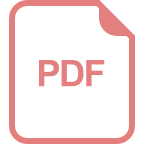
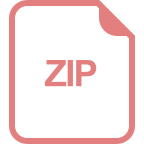
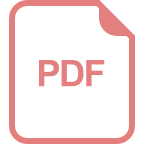
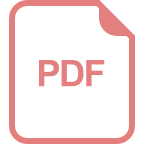
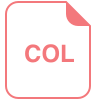
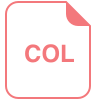





