Java编写图书管理系统的代码
时间: 2024-03-18 11:42:27 浏览: 48
以下是一个简单的 Java 图书管理系统示例:
1. Book 类:
```java
public class Book {
private String id;
private String name;
private String author;
private int price;
public Book(String id, String name, String author, int price) {
this.id = id;
this.name = name;
this.author = author;
this.price = price;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public int getPrice() {
return price;
}
}
```
2. BookList 类:
```java
import java.util.ArrayList;
public class BookList {
private ArrayList<Book> books;
public BookList() {
books = new ArrayList<Book>();
}
public void add(Book book) {
books.add(book);
}
public void remove(Book book) {
books.remove(book);
}
public ArrayList<Book> searchByName(String name) {
ArrayList<Book> result = new ArrayList<Book>();
for (Book book : books) {
if (book.getName().equals(name)) {
result.add(book);
}
}
return result;
}
public ArrayList<Book> searchByAuthor(String author) {
ArrayList<Book> result = new ArrayList<Book>();
for (Book book : books) {
if (book.getAuthor().equals(author)) {
result.add(book);
}
}
return result;
}
public ArrayList<Book> getAll() {
return books;
}
}
```
3. Library 类:
```java
import java.util.Scanner;
public class Library {
private BookList bookList;
public Library() {
bookList = new BookList();
}
public void addBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入书籍编号:");
String id = scanner.nextLine();
System.out.print("请输入书籍名称:");
String name = scanner.nextLine();
System.out.print("请输入书籍作者:");
String author = scanner.nextLine();
System.out.print("请输入书籍价格:");
int price = scanner.nextInt();
scanner.nextLine();
Book book = new Book(id, name, author, price);
bookList.add(book);
System.out.println("添加成功!");
}
public void removeBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入要删除的书籍编号:");
String id = scanner.nextLine();
ArrayList<Book> books = bookList.getAll();
for (Book book : books) {
if (book.getId().equals(id)) {
bookList.remove(book);
System.out.println("删除成功!");
return;
}
}
System.out.println("没有找到编号为 " + id + " 的书籍!");
}
public void searchBookByName() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入要查询的书籍名称:");
String name = scanner.nextLine();
ArrayList<Book> result = bookList.searchByName(name);
if (result.size() == 0) {
System.out.println("没有找到书名为 " + name + " 的书籍!");
} else {
System.out.println("查询结果:");
for (Book book : result) {
System.out.println(book.getId() + " " + book.getName() + " " + book.getAuthor() + " " + book.getPrice());
}
}
}
public void searchBookByAuthor() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入要查询的书籍作者:");
String author = scanner.nextLine();
ArrayList<Book> result = bookList.searchByAuthor(author);
if (result.size() == 0) {
System.out.println("没有找到作者为 " + author + " 的书籍!");
} else {
System.out.println("查询结果:");
for (Book book : result) {
System.out.println(book.getId() + " " + book.getName() + " " + book.getAuthor() + " " + book.getPrice());
}
}
}
public void printMenu() {
System.out.println("1. 添加书籍");
System.out.println("2. 删除书籍");
System.out.println("3. 按书名查询");
System.out.println("4. 按作者查询");
System.out.println("5. 退出系统");
}
public void run() {
Scanner scanner = new Scanner(System.in);
while (true) {
printMenu();
System.out.print("请选择操作:");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
addBook();
break;
case 2:
removeBook();
break;
case 3:
searchBookByName();
break;
case 4:
searchBookByAuthor();
break;
case 5:
System.out.println("谢谢使用!");
return;
default:
System.out.println("无效操作,请重新选择!");
}
System.out.println();
}
}
}
```
4. Main 类:
```java
public class Main {
public static void main(String[] args) {
Library library = new Library();
library.run();
}
}
```
运行以上代码后,你可以通过控制台输入来添加、删除、查询图书信息。你可以根据需求修改代码实现你自己的图书管理系统。
阅读全文
相关推荐
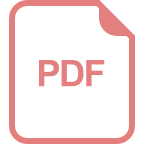
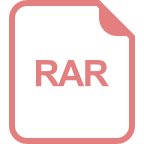
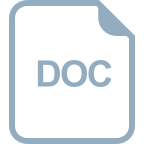
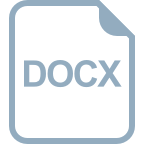
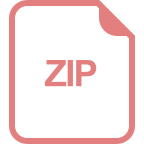
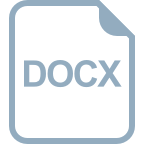
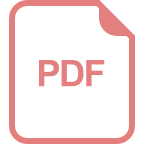
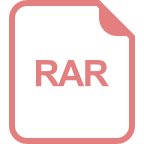
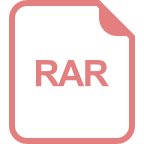
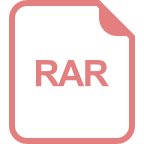
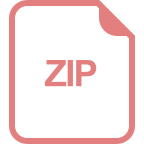
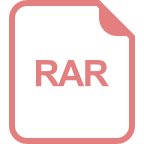
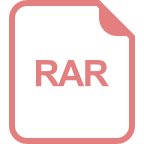
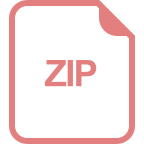
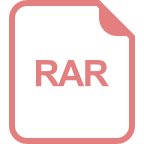
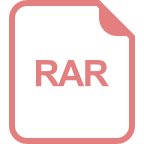
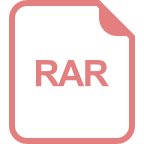