基于SpringBoot的请假系统,使用echarts的堆叠柱状图可视化1-12月的不同类型的请假次数统计,持久层调用数据,并使用model封装
时间: 2024-02-11 14:07:19 浏览: 144
好的,您的问题是关于基于SpringBoot的请假系统,使用echarts的堆叠柱状图可视化1-12月的不同类型的请假次数统计,持久层调用数据,并使用model封装。
首先,您可以在SpringBoot中定义一个请假实体类,包含请假人员姓名、请假开始时间、请假结束时间、请假类型等属性,并使用JPA或Mybatis等框架进行持久化操作。然后,您可以在SpringBoot中使用echarts进行可视化展示。首先,在前端页面引入echarts库,并创建一个堆叠柱状图实例。然后,使用Ajax请求后端接口获取数据库中请假数据,并将数据传入堆叠柱状图中进行展示。
接下来,您需要进行数据处理,将不同类型的请假次数统计数据封装到一个model中。例如:
```
// 后端SpringBoot代码
@RestController
@RequestMapping("/leave")
public class LeaveController {
@Autowired
private LeaveRepository leaveRepository;
@GetMapping("/statistics")
public LeaveStatisticsModel getLeaveStatistics() {
LeaveStatisticsModel model = new LeaveStatisticsModel();
List<Object[]> list = leaveRepository.getLeaveStatistics();
for (Object[] obj : list) {
int month = Integer.parseInt(obj[0].toString());
int count = Integer.parseInt(obj[1].toString());
String type = obj[2].toString();
switch (type) {
case "事假":
model.getPersonalLeaveCount()[month - 1] = count;
break;
case "病假":
model.getSickLeaveCount()[month - 1] = count;
break;
case "年假":
model.getAnnualLeaveCount()[month - 1] = count;
break;
// 其他类型的请假统计
default:
break;
}
}
return model;
}
}
// model类
public class LeaveStatisticsModel {
private int[] personalLeaveCount = new int[12];
private int[] sickLeaveCount = new int[12];
private int[] annualLeaveCount = new int[12];
// 其他类型的请假统计数组
public int[] getPersonalLeaveCount() {
return personalLeaveCount;
}
public void setPersonalLeaveCount(int[] personalLeaveCount) {
this.personalLeaveCount = personalLeaveCount;
}
public int[] getSickLeaveCount() {
return sickLeaveCount;
}
public void setSickLeaveCount(int[] sickLeaveCount) {
this.sickLeaveCount = sickLeaveCount;
}
public int[] getAnnualLeaveCount() {
return annualLeaveCount;
}
public void setAnnualLeaveCount(int[] annualLeaveCount) {
this.annualLeaveCount = annualLeaveCount;
}
// 其他类型的请假统计数组的getter和setter方法
}
```
在前端JS代码中,您需要将model中的数据进行拼接,然后传入堆叠柱状图中进行展示。例如:
```
// 前端JS代码
var myChart = echarts.init(document.getElementById('leave-chart'));
$.ajax({
url: '/leave/statistics',
type: 'GET',
dataType: 'json',
success: function(data) {
// 数据处理
var legendData = ['事假', '病假', '年假']; // 其他类型的请假统计
var xData = ['1月', '2月', '3月', '4月', '5月', '6月', '7月', '8月', '9月', '10月', '11月', '12月'];
var seriesData = [];
for (var i = 0; i < legendData.length; i++) {
var item = {
name: legendData[i],
type: 'bar',
stack: '总量',
data: data[legendData[i].toLowerCase() + 'LeaveCount']
};
seriesData.push(item);
}
// 堆叠柱状图配置
var option = {
legend: {
data: legendData
},
xAxis: {
type: 'category',
data: xData
},
yAxis: {
type: 'value'
},
series: seriesData
};
// 使用刚指定的配置项和数据显示图表。
myChart.setOption(option);
}
});
```
以上就是基于SpringBoot的请假系统,使用echarts的堆叠柱状图可视化1-12月的不同类型的请假次数统计,持久层调用数据,并使用model封装的实现方式。
阅读全文
相关推荐
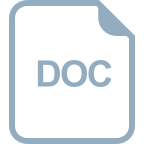
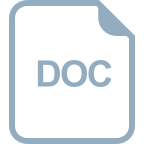
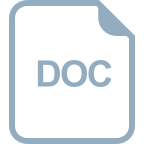



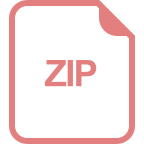
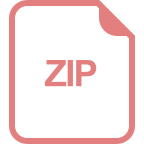
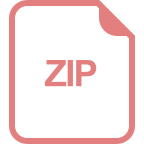
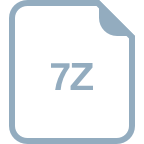
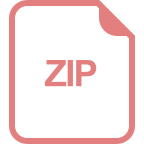
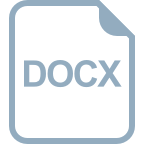
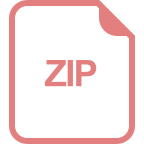
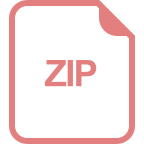
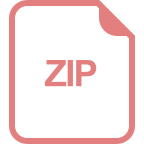
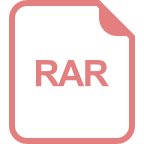
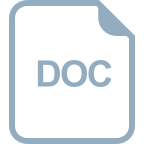