使用Apache Curator 结合 JUnit4 通过创建单元测试方法完成以下任务: 创建一个客户端, 连接到ZooKeeper服务器, 指定namespace为niit 为节点/foo/bar设置NodeCache缓存并添加监听, 监听事件触发则打印缓存数据相关信息 分别演示创建(递归), 修改, 删除(递归)/foo/bar节点 观察日志输出的详细步骤应该怎么操作,请分别举例说明操作步骤
时间: 2024-01-21 14:16:46 浏览: 75
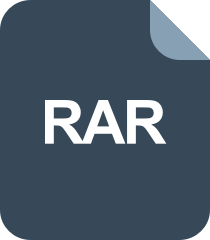
zookeeper 使用 Curator 示例监听、分布式锁
以下是使用Apache Curator 结合 JUnit4 创建单元测试方法来完成上述任务的步骤说明:
1. 首先,在测试类上添加注解 `@RunWith(CuratorTestRunner.class)`,因为我们要使用CuratorTestRunner来运行测试方法。
2. 接着,在测试类中定义一个ZooKeeper客户端,并在 `@Before` 方法上初始化该客户端连接到ZooKeeper服务器,并指定namespace为"niit":
```java
private static final String ZK_TEST_SERVER = "localhost:2181";
private static final int SESSION_TIMEOUT_MS = 5000;
private static final String NAMESPACE = "niit";
private CuratorFramework client;
@Before
public void setup() {
client = CuratorFrameworkFactory.builder()
.connectString(ZK_TEST_SERVER)
.sessionTimeoutMs(SESSION_TIMEOUT_MS)
.namespace(NAMESPACE)
.retryPolicy(new RetryNTimes(3, 1000))
.build();
client.start();
}
```
3. 接下来,我们需要创建一个节点并添加NodeCache缓存并添加监听器。在 `@Test` 方法中,我们可以使用 `client.create()` 方法来创建节点,并使用 `NodeCache` 类来监听节点变化。具体代码如下:
```java
@Test
public void testCreateNodeWithCache() throws Exception {
String path = "/foo/bar";
byte[] data = "Hello, World!".getBytes();
client.create().creatingParentsIfNeeded().forPath(path, data);
NodeCache nodeCache = new NodeCache(client, path);
nodeCache.start(true);
nodeCache.getListenable().addListener(() -> {
ChildData childData = nodeCache.getCurrentData();
if (childData != null) {
System.out.println("Node changed. Path: " + childData.getPath() + ", Data: " + new String(childData.getData()));
}
});
Thread.sleep(1000); // Wait for the listener to be registered
client.setData().forPath(path, "Hello, Curator!".getBytes());
client.delete().deletingChildrenIfNeeded().forPath(path);
nodeCache.close();
}
```
以上代码中,我们创建了路径为 `/foo/bar` 的节点,并且设置了节点数据为 "Hello, World!"。然后,我们使用 `NodeCache` 类创建了一个节点缓存,并添加了一个监听器,用于监听节点变化。在监听器中,我们使用 `getCurrentData()` 方法获取当前节点的信息,并打印出来。
接下来,我们修改节点 `/foo/bar` 的数据为 "Hello, Curator!",然后删除该节点及其所有子节点。这样就会触发监听器的回调函数,输出节点变化信息。
4. 最后,在 `@After` 方法中关闭ZooKeeper客户端连接:
```java
@After
public void tearDown() {
if (client != null) {
client.close();
}
}
```
至此,我们就完成了使用Apache Curator结合JUnit4创建单元测试方法来监听ZooKeeper节点变化的任务。
阅读全文
相关推荐
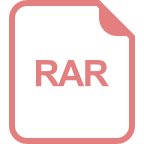
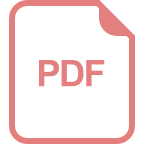

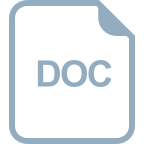
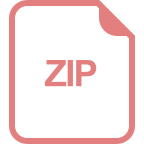
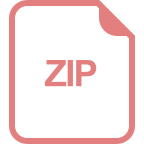
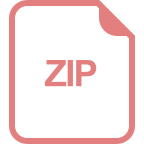
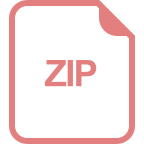
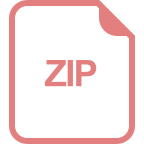
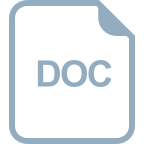
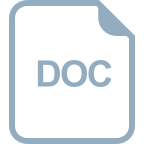
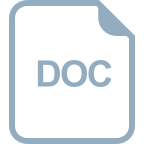
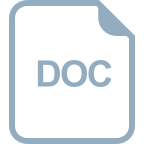
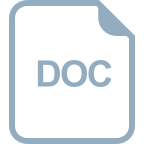

