基于stm32 7孔oled显示中文代码
时间: 2023-08-04 15:01:06 浏览: 163
要在STM32上使用7孔OLED显示中文,需要以下几个步骤:
1. 接线:将OLED模块的VCC连接到STM32的3.3V电源引脚,GND连接到GND引脚,SCL连接到STM32的I2C SCL引脚,SDA连接到STM32的I2C SDA引脚。
2. 在STM32上配置I2C总线:使用STM32的I2C库来配置I2C总线,设置I2C速度和地址。确保STM32正确连接到OLED模块。
3. 引入和配置字库:通过从网上下载合适的中文字库文件,并将其添加到STM32工程中。然后在代码中引入字库并正确设置其编码格式。
4. 编写显示中文的代码:使用OLED库和字库,编写代码来显示中文字符。这包括转换字符编码、查找字符对应的字模和逐个点亮OLED屏上的像素来显示字符。
5. 测试和调试:将代码烧入STM32板,将OLED模块连接到STM32,然后上传代码并检查OLED上是否正确显示中文字符。根据需要进行调试和优化。
需要注意的是,这只是一个简单的步骤指南,具体实现还需要根据使用的OLED模块和STM32型号进行调整。另外,还需要根据具体情况选择适合的OLED库和字库。
相关问题
基于STM32编写OLED波形显示代码
好的,我明白了。关于您的问题,我可以提供一个简单的代码,用于在OLED屏幕上显示波形。
首先,您需要确保已经在STM32上正确配置了OLED屏幕,并且已经将屏幕的驱动程序与STM32进行了连接。
接下来,您需要编写代码,获取波形数据,将其转换为OLED屏幕的像素,并将其显示在屏幕上。
以下是一个简单的示例代码,用于在OLED屏幕上显示波形:
```c
#include <stdint.h>
#include "oled.h"
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
void draw_waveform(uint16_t* waveform_data, uint16_t waveform_length) {
// calculate scaling factors
uint16_t max_value = 0;
for (int i = 0; i < waveform_length; i++) {
if (waveform_data[i] > max_value) {
max_value = waveform_data[i];
}
}
float x_scale = SCREEN_WIDTH / (float)(waveform_length - 1);
float y_scale = SCREEN_HEIGHT / (float)(max_value - 1);
// draw waveform on OLED screen
oled_clear();
for (int i = 0; i < waveform_length - 1; i++) {
uint8_t x1 = x_scale * i;
uint8_t x2 = x_scale * (i + 1);
uint8_t y1 = SCREEN_HEIGHT - (waveform_data[i] * y_scale);
uint8_t y2 = SCREEN_HEIGHT - (waveform_data[i + 1] * y_scale);
oled_draw_line(x1, y1, x2, y2);
}
oled_update();
}
int main() {
// initialize OLED screen and waveform data
oled_init();
uint16_t waveform_data[] = { 10, 20, 30, 40, 50, 60, 70, 80, 90, 100 };
// draw waveform on OLED screen
draw_waveform(waveform_data, 10);
// loop forever
while (1) {
}
return 0;
}
```
这个代码只是一个简单的示例,您可能需要对其进行修改,以适应您的特定硬件和应用程序需求。同时,这个代码也只用于展示在OLED屏幕上绘制波形数据的基本思路。
基于stm32oled显示年月日的代码
以下是基于STM32和OLED显示屏的年月日显示代码:
```c
#include "stm32f10x.h"
#include "oled.h"
#include "stdlib.h"
#include "stdio.h"
#include "delay.h"
#include "iic.h"
#define I2C_Speed 400000
#define I2C1_SLAVE_ADDRESS7 0x78
u8 year,month,day,week;
u8 hour,minute,second;
void RTC_Configuration(void);
void I2C_Configuration(void);
void TIM_Configuration(void);
void NVIC_Configuration(void);
void OLED_Init(void);
void OLED_ShowStr(u8 x,u8 y, u8 ch[], u8 TextSize);
void OLED_ShowNum(u8 x,u8 y, u32 num, u8 len, u8 TextSize);
void OLED_ShowTime(u8 x,u8 y);
void OLED_ShowDate(u8 x,u8 y);
void OLED_ShowWeek(u8 x,u8 y);
int main(void)
{
NVIC_Configuration();
TIM_Configuration();
OLED_Init();
RTC_Configuration();
I2C_Configuration();
while(1)
{
OLED_ShowTime(0,0);
OLED_ShowDate(0,2);
OLED_ShowWeek(0,4);
}
}
void RTC_Configuration(void)
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR, ENABLE);
PWR_BackupAccessCmd(ENABLE);
RCC_LSEConfig(RCC_LSE_ON);
while (RCC_GetFlagStatus(RCC_FLAG_LSERDY) == RESET);
RCC_RTCCLKConfig(RCC_RTCCLKSource_LSE);
RCC_RTCCLKCmd(ENABLE);
RTC_WaitForSynchro();
RTC_WaitForLastTask();
RTC_SetPrescaler(32767);
RTC_WaitForLastTask();
RTC_ITConfig(RTC_IT_SEC, ENABLE);
RTC_WaitForLastTask();
}
void I2C_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
I2C_InitTypeDef I2C_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_I2C1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
I2C_DeInit(I2C1);
I2C_InitStructure.I2C_Mode = I2C_Mode_I2C;
I2C_InitStructure.I2C_DutyCycle = I2C_DutyCycle_2;
I2C_InitStructure.I2C_OwnAddress1 = I2C1_SLAVE_ADDRESS7;
I2C_InitStructure.I2C_Ack = I2C_Ack_Enable;
I2C_InitStructure.I2C_AcknowledgedAddress = I2C_AcknowledgedAddress_7bit;
I2C_InitStructure.I2C_ClockSpeed = I2C_Speed;
I2C_Init(I2C1, &I2C_InitStructure);
I2C_Cmd(I2C1, ENABLE);
}
void TIM_Configuration(void)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
TIM_TimeBaseStructure.TIM_Period = 999;
TIM_TimeBaseStructure.TIM_Prescaler = 7199;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
TIM_ITConfig(TIM2, TIM_IT_Update, ENABLE);
TIM_Cmd(TIM2, ENABLE);
NVIC_InitStructure.NVIC_IRQChannel = TIM2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
}
void NVIC_Configuration(void)
{
NVIC_InitTypeDef NVIC_InitStructure;
EXTI_InitTypeDef EXTI_InitStructure;
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2);
NVIC_InitStructure.NVIC_IRQChannel = RTC_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 2;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
EXTI_ClearITPendingBit(EXTI_Line17);
EXTI_InitStructure.EXTI_Line = EXTI_Line17;
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt;
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Rising;
EXTI_InitStructure.EXTI_LineCmd = ENABLE;
EXTI_Init(&EXTI_InitStructure);
}
void OLED_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_10 | GPIO_Pin_11;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOB, &GPIO_InitStructure);
OLED_InitGpio();
OLED_InitI2c();
OLED_InitDev();
OLED_Fill(0x00);
OLED_Refresh_Gram();
}
void OLED_ShowStr(u8 x,u8 y, u8 ch[], u8 TextSize)
{
u8 c = 0,i = 0,j = 0;
switch(TextSize)
{
case 1:
while (ch[j] != '\0')
{
c = ch[j] - 32;
if(x>128-6)
{
x=0;
y++;
}
OLED_ShowChar(x,y,c,TextSize);
x+=6;
j++;
}
break;
case 2:
while (ch[j] != '\0')
{
c = ch[j] - 32;
if(x>128-12)
{
x=0;
y++;
}
OLED_ShowChar(x,y,c,TextSize);
x+=12;
j++;
}
break;
}
}
void OLED_ShowNum(u8 x,u8 y, u32 num, u8 len, u8 TextSize)
{
u8 t,temp;
u8 enshow=0;
for(t=0;t<len;t++)
{
temp=(num/10^(len-t-1))%10;
if(enshow==0&&t<(len-1))
{
if(temp==0)
{
OLED_ShowChar(x+(TextSize/2)*t,y,' ',TextSize);
continue;
}
else enshow=1;
}
OLED_ShowChar(x+(TextSize/2)*t,y,temp+'0',TextSize);
}
}
void OLED_ShowTime(u8 x,u8 y)
{
RTC_GetTime(RTC_Format_BIN, &RTC_TimeStructure);
hour = RTC_TimeStructure.RTC_Hours;
minute = RTC_TimeStructure.RTC_Minutes;
second = RTC_TimeStructure.RTC_Seconds;
OLED_ShowNum(x,y,hour,2,1);
OLED_ShowChar(x+2*6,y,':',1);
OLED_ShowNum(x+3*6,y,minute,2,1);
OLED_ShowChar(x+5*6,y,':',1);
OLED_ShowNum(x+6*6,y,second,2,1);
}
void OLED_ShowDate(u8 x,u8 y)
{
RTC_GetDate(RTC_Format_BIN, &RTC_DateStructure);
year = RTC_DateStructure.RTC_Year;
month = RTC_DateStructure.RTC_Month;
day = RTC_DateStructure.RTC_Date;
OLED_ShowNum(x,y,year,4,1);
OLED_ShowChar(x+4*6,y,'/',1);
OLED_ShowNum(x+5*6,y,month,2,1);
OLED_ShowChar(x+7*6,y,'/',1);
OLED_ShowNum(x+8*6,y,day,2,1);
}
void OLED_ShowWeek(u8 x,u8 y)
{
RTC_GetDate(RTC_Format_BIN, &RTC_DateStructure);
week = RTC_DateStructure.RTC_WeekDay;
switch(week)
{
case 1:
OLED_ShowStr(x,y,"Monday",1);
break;
case 2:
OLED_ShowStr(x,y,"Tuesday",1);
break;
case 3:
OLED_ShowStr(x,y,"Wednesday",1);
break;
case 4:
OLED_ShowStr(x,y,"Thursday",1);
break;
case 5:
OLED_ShowStr(x,y,"Friday",1);
break;
case 6:
OLED_ShowStr(x,y,"Saturday",1);
break;
case 7:
OLED_ShowStr(x,y,"Sunday",1);
break;
}
}
```
请注意,这只是一个示例代码,实际使用时需要根据自己的硬件和软件环境进行适当的修改。同时需要注意的是,本代码仅提供参考,对于代码的正确性和安全性,需读者自行进行检验和验证。
阅读全文
相关推荐
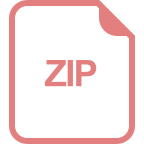
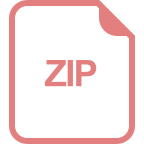
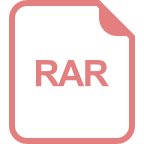
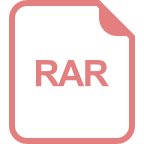
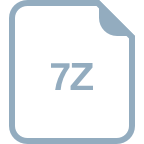
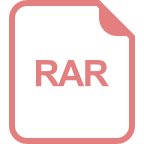
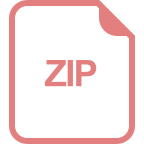
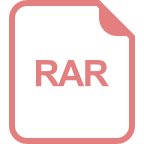



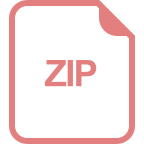
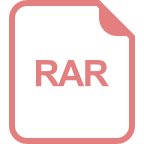
