我在C语言编写超市管理系统,以文件的形式储存结构体数据,我现在已经写好了增查功能,如何不用链表并用数据替换的形式写出删除功能
时间: 2023-05-27 13:03:26 浏览: 76
可以采用以下步骤实现不用链表并用数据替换的删除功能:
1. 定义一个结构体数组来存储超市商品信息,数组长度根据需要进行调整。
2. 编写一个函数来读取文件中的商品信息,将其存储到结构体数组中。
3. 编写一个函数来根据用户输入的商品编号或商品名称,查找商品在结构体数组中的位置,如果不存在就返回提示信息。
4. 编写一个函数来删除商品,即通过前面编写的查找函数找到商品在结构体数组中的位置,然后用后面的元素依次覆盖前面的元素,最后将数组长度减1。
5. 编写一个函数来保存更新后的商品信息到文件中。
下面是实现上述步骤的代码示例:
```c
#include <stdio.h>
#include <string.h>
#define MAX_PRODUCTS 100 // 最大商品数量
// 定义商品结构体
typedef struct {
int id; // 商品编号
char name[20]; // 商品名称
float price; // 商品单价
int stock; // 商品库存
} Product;
// 定义商品数组和当前商品数量
Product products[MAX_PRODUCTS];
int num_products = 0;
// 从文件中读取商品信息到数组中
void load_products()
{
FILE *fp = fopen("products.txt", "r");
if (fp != NULL) {
while (num_products < MAX_PRODUCTS && fscanf(fp, "%d %s %f %d",
&products[num_products].id, products[num_products].name,
&products[num_products].price, &products[num_products].stock) == 4) {
num_products++;
}
fclose(fp);
}
}
// 根据商品编号或名称查找商品的位置
int find_product(char *keyword)
{
int i;
for (i = 0; i < num_products; i++) {
if (strcmp(keyword, products[i].name) == 0 || atoi(keyword) == products[i].id) {
return i;
}
}
printf("Error: product not found.\n");
return -1;
}
// 删除商品
void delete_product(char *keyword)
{
int pos = find_product(keyword);
if (pos >= 0) {
// 用后面的元素依次覆盖前面的元素
int i;
for (i = pos + 1; i < num_products; i++) {
products[i - 1] = products[i];
}
// 数组长度减1
num_products--;
printf("Product deleted.\n");
}
}
// 保存更新后的商品信息到文件中
void save_products()
{
FILE *fp = fopen("products.txt", "w");
int i;
for (i = 0; i < num_products; i++) {
fprintf(fp, "%d %s %.2f %d\n", products[i].id, products[i].name,
products[i].price, products[i].stock);
}
fclose(fp);
}
int main()
{
load_products();
// 调用删除函数删除商品
char keyword[20];
printf("Enter the product ID or name you want to delete: ");
scanf("%s", keyword);
delete_product(keyword);
save_products();
return 0;
}
```
阅读全文
相关推荐
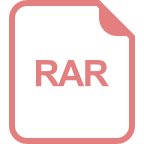
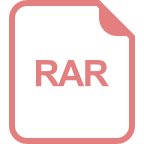
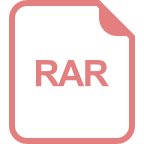
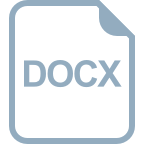
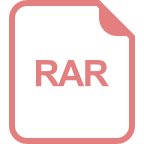
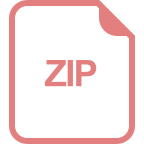
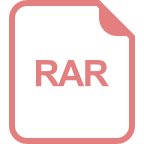
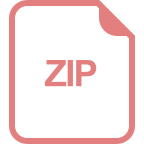
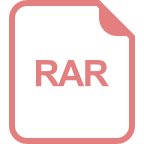
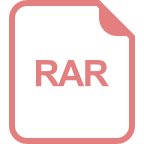
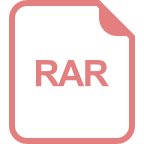
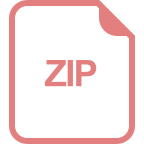
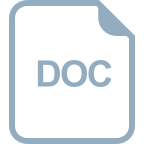
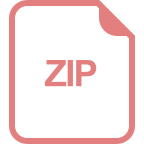
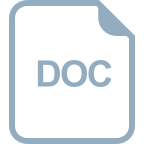
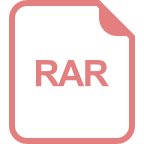
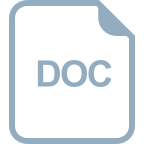