按如下规则对一个三位的正整数加密,各数位上的数都用加7的和除以10的余数取代,再把个位与百位交换。请根据加密规则进行解密。
时间: 2024-11-27 08:13:41 浏览: 5
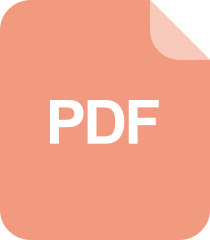
一种求正整数幂的高效算法详解
当然,我们可以编写一个简单的C++程序来实现这个加密和解密过程。首先,我们需要定义一个函数来进行加密,然后另一个函数来进行解密。
加密函数(Encrypt):
```cpp
int encrypt(int num) {
int remainder = (num % 10) + 7;
if (remainder >= 10) {
remainder -= 10;
}
// 获取十位和百位
int tens = (num / 10) % 10;
int hundreds = num / 100;
// 交换个位和百位
int encrypted_num = hundreds * 100 + tens * 10 + remainder;
return encrypted_num;
}
```
解密函数(Decrypt):
```cpp
int decrypt(int encrypted_num) {
// 分离个位、十位和百位
int remainder = encrypted_num % 10;
int tens = (encrypted_num / 10) % 10;
int hundreds = encrypted_num / 100;
// 重新组合并减去7后取模10,还原原始数值
int decrypted_num = (hundreds * 100 + tens * 10 - remainder) % 10;
return decrypted_num;
}
```
你可以像这样使用这两个函数:
```cpp
int main() {
int input_num, encrypted, decrypted;
std::cout << "请输入一个三位正整数:";
std::cin >> input_num;
encrypted = encrypt(input_num);
std::cout << "加密后的数字是:" << encrypted << std::endl;
decrypted = decrypt(encrypted);
std::cout << "解密后的数字是:" << decrypted << std::endl;
return 0;
}
```
运行上述代码,输入一个三位正整数,就可以看到加密和解密的结果了。
阅读全文
相关推荐
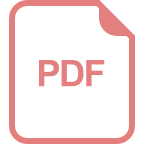
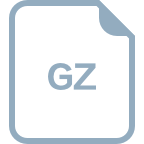













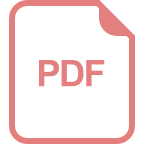
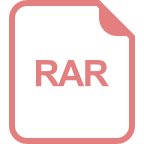
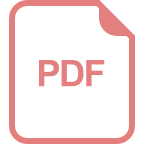