python收银系统
时间: 2023-07-16 07:14:23 浏览: 68
好的,一个简单的Python收银系统可以包含以下功能:
1. 用户输入商品信息和价格
2. 计算商品总价
3. 提供折扣和优惠券功能
4. 生成收据并打印
下面是一个简单的实现示例:
```python
# 收银系统
class Product:
def __init__(self, name, price):
self.name = name
self.price = price
class Cashier:
def __init__(self):
self.products = []
self.total_price = 0
self.discount = 0
self.coupon = 0
def add_product(self, product):
self.products.append(product)
self.total_price += product.price
def apply_discount(self, discount):
self.discount = discount
self.total_price *= (1 - discount)
def apply_coupon(self, coupon):
self.coupon = coupon
self.total_price -= coupon
def print_receipt(self):
print("Product\t\tPrice")
for product in self.products:
print(f"{product.name}\t\t{product.price}")
print(f"Total price:\t{self.total_price}")
print(f"Discount:\t{self.discount}")
print(f"Coupon:\t\t{self.coupon}")
print(f"Final price:\t{self.total_price - self.coupon}")
# 使用示例
cashier = Cashier()
# 添加商品
cashier.add_product(Product("Apple", 2.5))
cashier.add_product(Product("Banana", 1.5))
cashier.add_product(Product("Orange", 3.0))
# 计算总价
cashier.apply_discount(0.1)
cashier.apply_coupon(1.0)
# 打印收据
cashier.print_receipt()
```
这个示例实现了一个简单的收银系统,包含了添加商品、计算总价、打折和优惠券等功能,并能够生成收据并打印。你可以根据自己的需求进行修改和扩展。
相关推荐
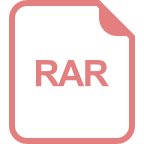














