【NumberFormatException异常】编写一个程序,提示用户输入两个整数,然后显示它们的和。用户输入错误时提示用户重新输入。 【ArrayIndexOutOfBoundsException异常】编写一个程序,创建一个由100个随机选取的整数构成的数组;提示用户输入数组下标,然后显示元素的值,如果指定的下标越界,显示消息out of bounds。 【OutOfMemoryError错误】编写一个程序,它能导致JVM抛出一个OutOfMemoryError,然后捕获并处理这个错误。
时间: 2024-02-09 16:10:32 浏览: 22
好的,我会回答你的问题。
对于第一个问题,你可以参考下面的代码实现:
```java
import java.util.Scanner;
public class AddTwoIntegers {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int num1 = 0, num2 = 0;
boolean validInput = false;
do {
try {
System.out.print("Enter the first integer: ");
num1 = Integer.parseInt(scanner.nextLine());
System.out.print("Enter the second integer: ");
num2 = Integer.parseInt(scanner.nextLine());
validInput = true;
} catch (NumberFormatException e) {
System.out.println("Invalid input. Please enter a valid integer.");
}
} while (!validInput);
int sum = num1 + num2;
System.out.println("The sum of the two integers is " + sum);
}
}
```
对于第二个问题,你可以参考下面的代码实现:
```java
import java.util.Random;
import java.util.Scanner;
public class ArrayIndexOutOfBounds {
public static void main(String[] args) {
Random random = new Random();
int[] arr = new int[100];
for (int i = 0; i < 100; i++) {
arr[i] = random.nextInt(100);
}
Scanner scanner = new Scanner(System.in);
boolean validInput = false;
do {
try {
System.out.print("Enter the index of the element you want to access (0-99): ");
int index = Integer.parseInt(scanner.nextLine());
if (index < 0 || index > 99) {
System.out.println("Invalid index. Please enter an index between 0 and 99.");
} else {
System.out.println("The element at index " + index + " is " + arr[index]);
validInput = true;
}
} catch (NumberFormatException e) {
System.out.println("Invalid input. Please enter a valid integer.");
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Out of bounds. Please enter an index between 0 and 99.");
}
} while (!validInput);
}
}
```
对于第三个问题,你可以参考下面的代码实现:
```java
import java.util.ArrayList;
import java.util.List;
public class OutOfMemoryErrorExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
try {
while (true) {
list.add(new String("This is a string."));
}
} catch (OutOfMemoryError e) {
System.out.println("Out of memory.");
}
}
}
```
这个程序会不断地往ArrayList中添加新的字符串对象,直到JVM抛出OutOfMemoryError。在catch块中,我们捕获这个错误并输出一条消息。
相关推荐
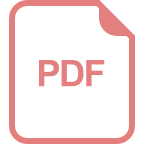
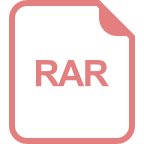
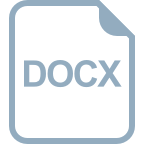















