下面的矩阵中包含 A B C D E F ABCDEF 六种字符,请问出现最多的字符出现了几次?python语言代码
时间: 2024-10-23 08:05:03 浏览: 6
为了找出给定矩阵中出现次数最多的字符及其出现次数,你可以遍历矩阵,统计每个字符的计数,并保持最大出现次数和对应的字符。下面是一个简单的Python代码示例:
```python
matrix = ["A", "B", "C", "D", "E", "F", "A", "B", "C", "D", "E", "F"]
char_count = {}
max_char = matrix[0]
max_count = 1
for char in matrix:
if char in char_count:
char_count[char] += 1
else:
char_count[char] = 1
if char_count[char] > max_count:
max_count = char_count[char]
max_char = char
most_frequent_char = max_char
occurrences = max_count
print(f"出现最多的字符 '{max_char}' 出现了 {occurrences} 次.")
```
这个代码首先创建了一个字典`char_count`来存储字符及其出现次数,然后通过迭代矩阵更新最大字符和次数。最后,它返回出现次数最多的字符以及相应的次数。
相关问题
用C语言编程。输入ABCDEF六个字符的权值,根据权值用哈夫曼算法建立哈夫曼树,并进行哈夫曼编码,输出每个字符对应的哈夫曼编码。
好的,让我来回答您的问题。下面是一个用C语言编写的实现哈夫曼编码的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CODE_LEN 256 // 哈夫曼编码最大长度
// 哈夫曼树节点结构体
typedef struct node {
int weight; // 权值
int parent, lchild, rchild; // 父节点、左右孩子节点下标
} node_t;
// 哈夫曼编码结构体
typedef struct code {
char ch; // 字符
char bits[MAX_CODE_LEN]; // 编码
} code_t;
// 选择两个节点中最小的节点
void select(node_t *nodes, int n, int *min1, int *min2) {
int i;
*min1 = *min2 = -1;
for (i = 0; i < n; i++) {
if (nodes[i].parent == -1) {
if (*min1 == -1 || nodes[i].weight < nodes[*min1].weight) {
*min2 = *min1;
*min1 = i;
} else if (*min2 == -1 || nodes[i].weight < nodes[*min2].weight) {
*min2 = i;
}
}
}
}
// 构建哈夫曼树
void huffman(node_t *nodes, int n) {
int i, j, min1, min2;
for (i = n; i < 2 * n - 1; i++) {
select(nodes, i, &min1, &min2);
nodes[min1].parent = nodes[min2].parent = i;
nodes[i].lchild = min1;
nodes[i].rchild = min2;
nodes[i].weight = nodes[min1].weight + nodes[min2].weight;
}
}
// 生成哈夫曼编码
void gen_huffman_code(node_t *nodes, int n, code_t *codes) {
char bits[MAX_CODE_LEN];
int i, j, parent, cur;
for (i = 0; i < n; i++) {
codes[i].ch = i + 'A';
bits[0] = '\0';
cur = i;
parent = nodes[cur].parent;
while (parent != -1) {
if (nodes[parent].lchild == cur) {
strcat(bits, "0");
} else {
strcat(bits, "1");
}
cur = parent;
parent = nodes[cur].parent;
}
// 需要翻转得到正确的哈夫曼编码
strrev(bits);
strcpy(codes[i].bits, bits);
}
}
// 输出哈夫曼编码表
void print_huffman_code_table(code_t *codes, int n) {
int i;
printf("Huffman Code Table:\n");
for (i = 0; i < n; i++) {
printf("%c: %s\n", codes[i].ch, codes[i].bits);
}
}
int main() {
int n = 6, i;
int weights[6] = {7, 5, 2, 4, 9, 8};
node_t nodes[2 * n - 1];
code_t codes[n];
memset(nodes, 0, sizeof(nodes));
memset(codes, 0, sizeof(codes));
for (i = 0; i < n; i++) {
nodes[i].weight = weights[i];
}
huffman(nodes, n);
gen_huffman_code(nodes, n, codes);
print_huffman_code_table(codes, n);
return 0;
}
```
上述代码中,我们先定义了一个`node_t`结构体表示哈夫曼树的节点,其中包括权值、父节点、左右孩子节点的下标信息;定义了一个`code_t`结构体表示哈夫曼编码,其中包括字符和编码信息。然后我们实现了选择两个节点中最小的节点的函数`select`、构建哈夫曼树的函数`huffman`、生成哈夫曼编码的函数`gen_huffman_code`和输出哈夫曼编码表的函数`print_huffman_code_table`。在`main`函数中,我们输入了6个字符的权值,然后调用上述函数进行哈夫曼编码,并输出哈夫曼编码表。
注意:上述代码中包含一些用于字符数组操作的辅助函数,比如`strcat`和`strrev`,可以访问这里了解详细实现。
编写一个C语言程序,由键盘输入两个字符串(假设第一个字符串必包含第二个字符串,如第一个字符串为ABCDEF,第二个为CDE,则CDE 包含在ABCDEF中)现要求编程输出第二字符串在第一行字符串中出现的位置。(如果第二个字符串在第一个字符串中出现多次,则以最前出现的为准)
以下是一个简单的C语言程序,可以实现以上要求:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char str1[100], str2[100];
int i, j, len1, len2, pos = -1;
printf("请输入第一个字符串:");
gets(str1);
printf("请输入第二个字符串:");
gets(str2);
len1 = strlen(str1);
len2 = strlen(str2);
for (i = 0; i <= len1 - len2; i++)
{
for (j = 0; j < len2; j++)
{
if (str1[i + j] != str2[j])
break;
}
if (j == len2)
{
pos = i;
break;
}
}
if (pos == -1)
printf("第二个字符串在第一个字符串中未出现!");
else
printf("第二个字符串在第一个字符串中的位置为:%d", pos);
return 0;
}
```
程序中先定义了两个字符串数组`str1`和`str2`,分别用于存储输入的两个字符串。然后使用`strlen`函数分别计算出两个字符串的长度,接着使用两个循环遍历第一个字符串,寻找第二个字符串的位置。其中,外层循环控制第一个字符串的起始位置,内层循环用于比较两个字符串的每个字符是否相同。如果相同,则说明第二个字符串在第一个字符串中出现了,记录下此时的起始位置并跳出循环。最后根据是否找到第二个字符串,输出不同的结果。
阅读全文
相关推荐
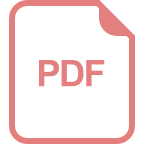
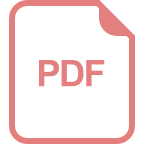
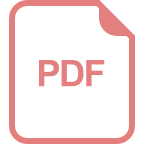













