matplotlib折线图如何将超过某个阈值的值标记出来
时间: 2023-06-01 12:05:54 浏览: 263
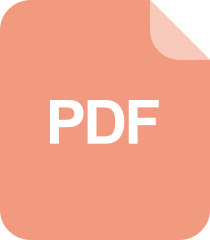
python matplotlib折线图样式实现过程

你可以使用Matplotlib库中的annotate()方法在绘制折线图时标记超过阈值的值。具体步骤如下:
1. 首先,计算超过阈值的数据点的索引或坐标。
2. 然后,使用annotate()方法在该点上添加标记文本或符号。
3. 最后,在图像中显示标记的数据点。
以下是一个示例代码片段,演示如何在折线图中标记超过阈值的值。
``` python
import matplotlib.pyplot as plt
# 数据集
x = [1, 2, 3, 4, 5]
y = [10, 20, 30, 35, 40]
# 阈值
threshold = 30
# 找到超过阈值的数据点
above_threshold = [i for i, val in enumerate(y) if val > threshold]
# 绘制折线图
plt.plot(x, y)
# 在超过阈值的数据点上添加标记
for i in above_threshold:
plt.annotate("Above", (x[i], y[i]))
# 显示图像
plt.show()
```
运行上述代码片段,你将获得一个折线图,其中超过阈值的值被标记为“Above”。
阅读全文
相关推荐
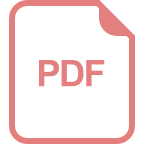
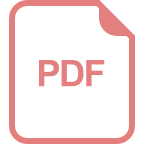
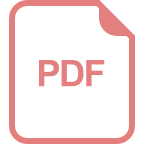
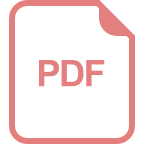
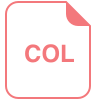
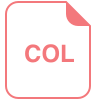
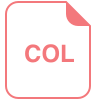
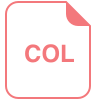
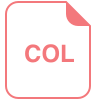
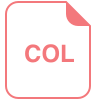

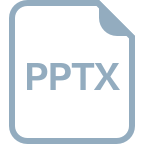
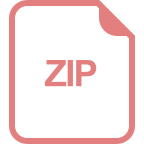
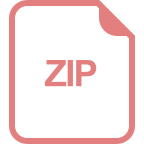
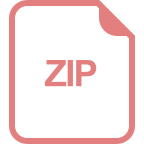