saveKeyword = (keyword) => { uni.getStorage({ key: 'OldKeys', success: res => { let OldKeys = JSON.parse(res.data) let findIndex = OldKeys.indexOf(keyword) if (findIndex == -1) { OldKeys.unshift(keyword) } else { OldKeys.splice(findIndex, 1) OldKeys.unshift(keyword) } //最多10个纪录 OldKeys.length > 10 && OldKeys.pop() uni.setStorage({ key: 'OldKeys', data: JSON.stringify(OldKeys) }) oldKeywordList = OldKeys //更新历史搜索 }, fail: e => { var OldKeys = [keyword] uni.setStorage({ key: 'OldKeys', data: JSON.stringify(OldKeys) }) oldKeywordList = OldKeys //更新历史搜索 } })换成vue3写法
时间: 2024-01-18 13:01:56 浏览: 94
在 Vue 3 中,可以使用 `localStorage` API 来进行本地存储。可以使用 `reactive` 和 `computed` 来实现响应式数据和计算属性,将方法定义在 `setup` 函数中。下面是修改后的代码:
```
<template>
<div>
<!-- 其他模板内容 -->
</div>
</template>
<script>
import { reactive, computed, onMounted } from 'vue'
export default {
setup() {
// 定义响应式数据
const state = reactive({
keywordList: [],
maxKeywordCount: 10,
defaultKeyword: 'default',
keyword: ''
})
// 计算属性
const oldKeywordList = computed(() => {
return state.keywordList.slice(1, state.maxKeywordCount + 1)
})
// 定义方法
function saveKeyword(keyword) {
let OldKeys = JSON.parse(localStorage.getItem('OldKeys')) || []
let findIndex = OldKeys.indexOf(keyword)
if (findIndex == -1) {
OldKeys.unshift(keyword)
} else {
OldKeys.splice(findIndex, 1)
OldKeys.unshift(keyword)
}
// 最多 maxKeywordCount 个记录
OldKeys.length > state.maxKeywordCount && OldKeys.pop()
localStorage.setItem('OldKeys', JSON.stringify(OldKeys))
state.keywordList = OldKeys // 更新历史搜索
}
// 生命周期函数
onMounted(() => {
// 从本地存储中获取历史搜索列表
let OldKeys = JSON.parse(localStorage.getItem('OldKeys')) || []
state.keywordList = OldKeys
})
// 返回响应式数据和方法
return { state, oldKeywordList, saveKeyword }
}
}
</script>
```
在 `setup` 函数中,通过 `reactive` 定义了响应式数据 `state`,包含了 `keywordList`、`maxKeywordCount`、`defaultKeyword` 和 `keyword` 四个属性。使用 `computed` 定义了响应式计算属性 `oldKeywordList`,用于计算最近搜索的关键字列表。定义了方法 `saveKeyword`,用于保存关键字到本地存储中,并更新历史搜索列表。使用 `onMounted` 生命周期函数,从本地存储中获取历史搜索列表并更新响应式数据 `state` 中的 `keywordList` 属性。最后,在返回的对象中返回了 `state`、`oldKeywordList` 和 `saveKeyword` 三个属性。
阅读全文
相关推荐
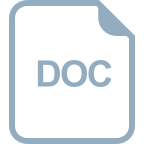
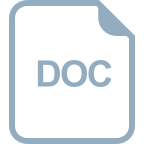
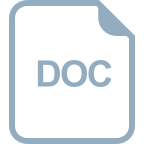















